mn create-app example.micronaut.micronautguide --build=gradle --lang=java
Access a database with JPA and Hibernate
Learn how to access a database with JPA and Hibernate using the Micronaut framework.
Authors: Iván López, Sergio del Amo
Micronaut Version: 4.9.1
1. Getting Started
In this guide, we will create a Micronaut application written in Java.
In this guide, we will write a Micronaut application that exposes some REST endpoints and stores data in a database using JPA and Hibernate.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE (e.g. IntelliJ IDEA)
-
JDK 21 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the application step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the Application
Create an application using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle with the Kotlin DSL is used as the build tool. If you don’t specify the --lang argument, Java is used as the language.If you don’t specify the --test argument, JUnit is used for Java and Kotlin, and Spock is used for Groovy.
|
The previous command creates a Micronaut application with the default package example.micronaut
in a directory named micronautguide
.
4.1. Data Source Dependencies
Add the following dependencies:
implementation("io.micronaut.sql:micronaut-hibernate-jpa") (1)
implementation("io.micronaut.data:micronaut-data-tx-hibernate") (2)
implementation("io.micronaut.sql:micronaut-jdbc-hikari") (3)
runtimeOnly("com.h2database:h2") (4)
1 | Configures Hibernate/JPA EntityManagerFactory beans. |
2 | Adds Micronaut Data Transaction Hibernate dependency. |
3 | Configures SQL DataSource instances using Hikari Connection Pool. |
4 | Add dependency to in-memory H2 Database. |
4.2. Data Source configuration
Define the data source in src/main/resources/application.properties
.
datasources.default.password=${JDBC_PASSWORD:""}
datasources.default.url=${JDBC_URL:`jdbc:h2:mem:default;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE`}
datasources.default.username=${JDBC_USER:sa}
datasources.default.driver-class-name=${JDBC_DRIVER:org.h2.Driver}
This way of defining the datasource properties means that we can externalize the configuration, for example for production environment, and also provide a default value for development. If the environment variables are not defined the Micronaut framework will use the default values. Also keep in mind that it is necessary to escape the : in the connection URL using backticks ` .
|
4.3. JPA configuration
Add the next snippet to src/main/resources/application.properties
to configure JPA:
jpa.default.properties.hibernate.hbm2ddl.auto=update
jpa.default.properties.hibernate.show_sql=true
4.4. Domain
Create the domain entities:
package example.micronaut.domain;
import com.fasterxml.jackson.annotation.JsonIgnore;
import io.micronaut.serde.annotation.Serdeable;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.Id;
import jakarta.persistence.OneToMany;
import jakarta.persistence.Table;
import jakarta.validation.constraints.NotNull;
import java.util.HashSet;
import java.util.Set;
import static jakarta.persistence.GenerationType.AUTO;
@Serdeable
@Entity
@Table(name = "genre")
public class Genre {
@Id
@GeneratedValue(strategy = AUTO)
private Long id;
@NotNull
@Column(name = "name", nullable = false, unique = true)
private String name;
@JsonIgnore
@OneToMany(mappedBy = "genre")
private Set<Book> books = new HashSet<>();
public Genre() {}
public Genre(@NotNull String name) {
this.name = name;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set<Book> getBooks() {
return books;
}
public void setBooks(Set<Book> books) {
this.books = books;
}
@Override
public String toString() {
return "Genre{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
The previous domain has a OneToMany
relationship with the domain Book
.
package example.micronaut.domain;
import io.micronaut.serde.annotation.Serdeable;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.Id;
import jakarta.persistence.ManyToOne;
import jakarta.persistence.Table;
import jakarta.validation.constraints.NotNull;
import static jakarta.persistence.GenerationType.AUTO;
@Serdeable
@Entity
@Table(name = "book")
public class Book {
@Id
@GeneratedValue(strategy = AUTO)
private Long id;
@NotNull
@Column(name = "name", nullable = false)
private String name;
@NotNull
@Column(name = "isbn", nullable = false)
private String isbn;
@ManyToOne
private Genre genre;
public Book() {}
public Book(@NotNull String isbn,
@NotNull String name,
Genre genre) {
this.isbn = isbn;
this.name = name;
this.genre = genre;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getIsbn() {
return isbn;
}
public void setIsbn(String isbn) {
this.isbn = isbn;
}
public Genre getGenre() {
return genre;
}
public void setGenre(Genre genre) {
this.genre = genre;
}
@Override
public String toString() {
return "Book{" +
"id=" + id +
", name='" + name + '\'' +
", isbn='" + isbn + '\'' +
", genre=" + genre +
'}';
}
}
4.5. Application Configuration
Create an interface to encapsulate the application configuration settings:
package example.micronaut;
public interface ApplicationConfiguration {
int getMax();
}
Like Spring Boot and Grails, in Micronaut applications you can create typesafe configuration by creating classes that are annotated with @ConfigurationProperties.
Create a ApplicationConfigurationProperties
class:
package example.micronaut;
import io.micronaut.context.annotation.ConfigurationProperties;
@ConfigurationProperties("application") (1)
public class ApplicationConfigurationProperties implements ApplicationConfiguration {
private final int DEFAULT_MAX = 10;
private int max = DEFAULT_MAX;
@Override
public int getMax() {
return max;
}
public void setMax(int max) {
this.max = max;
}
}
1 | The @ConfigurationProperties annotation takes the configuration prefix. |
You can override max
if you add to your src/main/resources/application.properties
:
application.max=50
4.6. Validation
Micronaut validation is built on the standard framework – JSR 380, also known as Bean Validation 2.0. Micronaut Validation has built-in support for validation of beans that are annotated with jakarta.validation
annotations.
To use Micronaut Validation, you need the following dependencies:
annotationProcessor("io.micronaut.validation:micronaut-validation-processor")
implementation("io.micronaut.validation:micronaut-validation")
Alternatively, you can use Micronaut Hibernate Validator, which uses Hibernate Validator; a reference implementation of the validation API.
4.7. Repository Access
Next, create a repository interface to define the operations to access the database.
package example.micronaut;
import example.micronaut.domain.Genre;
import jakarta.validation.constraints.NotBlank;
import jakarta.validation.constraints.NotNull;
import java.util.List;
import java.util.Optional;
public interface GenreRepository {
Optional<Genre> findById(long id);
Genre save(@NotBlank String name);
Genre saveWithException(@NotBlank String name);
void deleteById(long id);
List<Genre> findAll(@NotNull SortingAndOrderArguments args);
int update(long id, @NotBlank String name);
}
To mark the transaction demarcations, we use the Jakarta jakarta.transaction.Transactional annotation.
Write the implementation:
package example.micronaut;
import example.micronaut.domain.Genre;
import io.micronaut.transaction.annotation.ReadOnly;
import io.micronaut.transaction.annotation.Transactional;
import jakarta.inject.Singleton;
import jakarta.persistence.EntityManager;
import jakarta.persistence.PersistenceException;
import jakarta.persistence.TypedQuery;
import jakarta.validation.constraints.NotBlank;
import jakarta.validation.constraints.NotNull;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
@Singleton (1)
public class GenreRepositoryImpl implements GenreRepository {
private static final List<String> VALID_PROPERTY_NAMES = Arrays.asList("id", "name");
private final EntityManager entityManager; (2)
private final ApplicationConfiguration applicationConfiguration;
public GenreRepositoryImpl(EntityManager entityManager, (2)
ApplicationConfiguration applicationConfiguration) {
this.entityManager = entityManager;
this.applicationConfiguration = applicationConfiguration;
}
@Override
@ReadOnly (3)
public Optional<Genre> findById(long id) {
return Optional.ofNullable(entityManager.find(Genre.class, id));
}
@Override
@Transactional (4)
public Genre save(@NotBlank String name) {
Genre genre = new Genre(name);
entityManager.persist(genre);
return genre;
}
@Override
@Transactional (4)
public void deleteById(long id) {
findById(id).ifPresent(entityManager::remove);
}
@ReadOnly (3)
public List<Genre> findAll(@NotNull SortingAndOrderArguments args) {
String qlString = "SELECT g FROM Genre as g";
if (args.order() != null && args.sort() != null && VALID_PROPERTY_NAMES.contains(args.sort())) {
qlString += " ORDER BY g." + args.sort() + ' ' + args.order().toLowerCase();
}
TypedQuery<Genre> query = entityManager.createQuery(qlString, Genre.class);
query.setMaxResults(args.max() != null ? args.max() : applicationConfiguration.getMax());
if (args.offset() != null) {
query.setFirstResult(args.offset());
}
return query.getResultList();
}
@Override
@Transactional (4)
public int update(long id, @NotBlank String name) {
return entityManager.createQuery("UPDATE Genre g SET name = :name where id = :id")
.setParameter("name", name)
.setParameter("id", id)
.executeUpdate();
}
@Override
@Transactional (4)
public Genre saveWithException(@NotBlank String name) {
save(name);
throw new PersistenceException();
}
}
1 | Use jakarta.inject.Singleton to designate a class as a singleton. |
2 | Easily inject an EntityManager . |
3 | All database access needs to be wrapped inside a transaction. As the method only reads data from the database, annotate it with @ReadOnly . |
4 | This method modifies the database, thus it is annotated with @Transactional . |
When you use the Micronaut Data annotation processor, the framework maps the jakarta.transaction.Transactional annotation to io.micronaut.transaction.annotation.Transactional . In the previous code sample, we must use Micronaut @Transactional /@ReadOnly annotations, and we cannot use jakarta.transaction annotations since we don’t use Micronaut Data in this tutorial.
|
4.8. Controller
Create two classes to encapsulate Save and Update operations:
package example.micronaut;
import io.micronaut.serde.annotation.Serdeable;
import jakarta.validation.constraints.NotBlank;
@Serdeable (1)
public class GenreSaveCommand {
@NotBlank
private String name;
public GenreSaveCommand(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
1 | Declare the @Serdeable annotation at the type level in your source code to allow the type to be serialized or deserialized. |
package example.micronaut;
import io.micronaut.serde.annotation.Serdeable;
import jakarta.validation.constraints.NotBlank;
@Serdeable
public class GenreUpdateCommand {
private long id;
@NotBlank
private String name;
public GenreUpdateCommand(long id, String name) {
this.id = id;
this.name = name;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Create a POJO to encapsulate Sorting and Pagination:
package example.micronaut;
import io.micronaut.core.annotation.Nullable;
import io.micronaut.serde.annotation.Serdeable;
import jakarta.validation.constraints.Pattern;
import jakarta.validation.constraints.Positive;
import jakarta.validation.constraints.PositiveOrZero;
@Serdeable
public record SortingAndOrderArguments(
@Nullable
@PositiveOrZero (1)
Integer offset,
@Nullable
@Positive (1)
Integer max,
@Nullable
@Pattern(regexp = "id|name") (1)
String sort,
@Nullable
@Pattern(regexp = "asc|ASC|desc|DESC") (1)
String order
) {
}
1 | Use jakarta.validation.constraints Constraints to ensure the incoming data matches your expectations. |
Create GenreController
, a controller which exposes a resource with the common CRUD operations:
package example.micronaut;
import example.micronaut.domain.Genre;
import io.micronaut.http.HttpResponse;
import io.micronaut.http.annotation.Body;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Delete;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.Post;
import io.micronaut.http.annotation.Put;
import io.micronaut.scheduling.TaskExecutors;
import io.micronaut.scheduling.annotation.ExecuteOn;
import jakarta.persistence.PersistenceException;
import jakarta.validation.Valid;
import java.net.URI;
import java.util.List;
import static io.micronaut.http.HttpHeaders.LOCATION;
@ExecuteOn(TaskExecutors.BLOCKING) (1)
@Controller("/genres") (2)
class GenreController {
private final GenreRepository genreRepository;
GenreController(GenreRepository genreRepository) { (3)
this.genreRepository = genreRepository;
}
@Get("/{id}") (4)
Genre show(Long id) {
return genreRepository
.findById(id)
.orElse(null); (5)
}
@Put (6)
HttpResponse<?> update(@Body @Valid GenreUpdateCommand command) { (7)
int numberOfEntitiesUpdated = genreRepository.update(command.getId(), command.getName());
return HttpResponse
.noContent()
.header(LOCATION, location(command.getId()).getPath()); (8)
}
@Get(value = "/list{?args*}") (9)
List<Genre> list(@Valid SortingAndOrderArguments args) {
return genreRepository.findAll(args);
}
@Post (10)
HttpResponse<Genre> save(@Body @Valid GenreSaveCommand cmd) {
Genre genre = genreRepository.save(cmd.getName());
return HttpResponse
.created(genre)
.headers(headers -> headers.location(location(genre.getId())));
}
@Post("/ex") (11)
HttpResponse<Genre> saveExceptions(@Body @Valid GenreSaveCommand cmd) {
try {
Genre genre = genreRepository.saveWithException(cmd.getName());
return HttpResponse
.created(genre)
.headers(headers -> headers.location(location(genre.getId())));
} catch(PersistenceException e) {
return HttpResponse.noContent();
}
}
@Delete("/{id}") (12)
HttpResponse<?> delete(Long id) {
genreRepository.deleteById(id);
return HttpResponse.noContent();
}
private URI location(Long id) {
return URI.create("/genres/" + id);
}
}
1 | It is critical that any blocking I/O operations (such as fetching the data from the database) are offloaded to a separate thread pool that does not block the Event loop. |
2 | The class is defined as a controller with the @Controller annotation mapped to the path /genres . |
3 | Constructor injection. |
4 | Maps a GET request to /genres/{id} which attempts to show a genre. This illustrates the use of a URL path variable. |
5 | Returning null when the genre doesn’t exist makes the Micronaut framework respond with 404 (not found). |
6 | Maps a PUT request to /genres which attempts to update a genre. |
7 | Add @Valid to any method parameter which requires validation. Use a POJO supplied as a JSON payload in the request to populate command. |
8 | It is easy to add custom headers to the response. |
9 | Maps a GET request to /genres which returns a list of genres. This mapping illustrates URL parameters being mapped to a single POJO. |
10 | Maps a POST request to /genres which attempts to save a genre. |
11 | Maps a POST request to /ex which generates an exception. |
12 | Maps a DELETE request to /genres/{id} which attempts to remove a genre. This illustrates the use of a URL path variable. |
4.9. Writing Tests
Create a test to verify the CRUD operations:
package example.micronaut;
import example.micronaut.domain.Genre;
import io.micronaut.core.type.Argument;
import io.micronaut.http.HttpRequest;
import io.micronaut.http.HttpResponse;
import io.micronaut.http.client.BlockingHttpClient;
import io.micronaut.http.client.HttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.http.client.exceptions.HttpClientResponseException;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import jakarta.inject.Inject;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import java.util.ArrayList;
import java.util.List;
import static io.micronaut.http.HttpHeaders.LOCATION;
import static io.micronaut.http.HttpStatus.BAD_REQUEST;
import static io.micronaut.http.HttpStatus.CREATED;
import static io.micronaut.http.HttpStatus.NOT_FOUND;
import static io.micronaut.http.HttpStatus.NO_CONTENT;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertThrows;
@MicronautTest (1)
class GenreControllerTest {
private BlockingHttpClient blockingClient;
@Inject
@Client("/")
HttpClient client; (2)
@BeforeEach
void setup() {
blockingClient = client.toBlocking();
}
@Test
void supplyAnInvalidOrderTriggersValidationFailure() {
HttpClientResponseException thrown = assertThrows(HttpClientResponseException.class, () ->
blockingClient.exchange(HttpRequest.GET("/genres/list?order=foo"))
);
assertNotNull(thrown.getResponse());
assertEquals(BAD_REQUEST, thrown.getStatus());
}
@Test
void testFindNonExistingGenreReturns404() {
HttpClientResponseException thrown = assertThrows(HttpClientResponseException.class, () ->
blockingClient.exchange(HttpRequest.GET("/genres/99"))
);
assertNotNull(thrown.getResponse());
assertEquals(NOT_FOUND, thrown.getStatus());
}
@Test
void testGenreCrudOperations() {
List<Long> genreIds = new ArrayList<>();
HttpRequest<?> request = HttpRequest.POST("/genres", new GenreSaveCommand("DevOps")); (3)
HttpResponse<?> response = blockingClient.exchange(request);
genreIds.add(entityId(response));
assertEquals(CREATED, response.getStatus());
request = HttpRequest.POST("/genres", new GenreSaveCommand("Microservices")); (3)
response = blockingClient.exchange(request);
assertEquals(CREATED, response.getStatus());
Long id = entityId(response);
genreIds.add(id);
request = HttpRequest.GET("/genres/" + id);
Genre genre = blockingClient.retrieve(request, Genre.class); (4)
assertEquals("Microservices", genre.getName());
request = HttpRequest.PUT("/genres", new GenreUpdateCommand(id, "Micro-services"));
response = blockingClient.exchange(request); (5)
assertEquals(NO_CONTENT, response.getStatus());
request = HttpRequest.GET("/genres/" + id);
genre = blockingClient.retrieve(request, Genre.class);
assertEquals("Micro-services", genre.getName());
request = HttpRequest.GET("/genres/list");
List<Genre> genres = blockingClient.retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(2, genres.size());
request = HttpRequest.POST("/genres/ex", new GenreSaveCommand("Microservices")); (3)
response = blockingClient.exchange(request);
assertEquals(NO_CONTENT, response.getStatus());
request = HttpRequest.GET("/genres/list");
genres = blockingClient.retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(2, genres.size());
request = HttpRequest.GET("/genres/list?max=1");
genres = blockingClient.retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(1, genres.size());
assertEquals("DevOps", genres.get(0).getName());
request = HttpRequest.GET("/genres/list?max=1&order=desc&sort=name");
genres = blockingClient.retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(1, genres.size());
assertEquals("Micro-services", genres.get(0).getName());
request = HttpRequest.GET("/genres/list?max=1&offset=10");
genres = blockingClient.retrieve(request, Argument.of(List.class, Genre.class));
assertEquals(0, genres.size());
// cleanup:
for (Long genreId : genreIds) {
request = HttpRequest.DELETE("/genres/" + genreId);
response = blockingClient.exchange(request);
assertEquals(NO_CONTENT, response.getStatus());
}
}
private Long entityId(HttpResponse response) {
String path = "/genres/";
String value = response.header(LOCATION);
if (value == null) {
return null;
}
int index = value.indexOf(path);
if (index != -1) {
return Long.valueOf(value.substring(index + path.length()));
}
return null;
}
}
1 | Annotate the class with @MicronautTest so the Micronaut framework will initialize the application context and the embedded server. More info. |
2 | Inject the HttpClient bean and point it to the embedded server. |
3 | Creating HTTP Requests is easy thanks to the Micronaut framework fluid API. |
4 | If you care just about the object in the response use retrieve . |
5 | Sometimes, receiving just the object is not enough and you need information about the response. In this case, instead of retrieve you should use the exchange method. |
5. Testing the Application
To run the tests:
./gradlew test
Then open build/reports/tests/test/index.html
in a browser to see the results.
6. Running the Application
To run the application, use the ./gradlew run
command, which starts the application on port 8080.
7. Using PostgreSQL
When running on production you want to use a real database instead of using H2. Let’s explain how to use PostgreSQL.
After installing Docker, execute the following command to run a PostgreSQL container:
docker run -it --rm \
-p 5432:5432 \
-e POSTGRES_USER=dbuser \
-e POSTGRES_PASSWORD=theSecretPassword \
-e POSTGRES_DB=micronaut \
postgres:11.5-alpine
Add PostgreSQL driver dependency:
runtimeOnly("org.postgresql:postgresql")
To use PostgreSQL, set up several environment variables which match those defined in application.properties
:
export JDBC_URL=jdbc:postgresql://localhost:5432/micronaut
export JDBC_USER=dbuser
export JDBC_PASSWORD=theSecretPassword
export JDBC_DRIVER=org.postgresql.Driver
Run the application again. If you look at the output you can see that the application uses PostgreSQL:
..
...
08:40:02.746 [main] INFO org.hibernate.dialect.Dialect - HHH000400: Using dialect: org.hibernate.dialect.PostgreSQL10Dialect
....
Connect to your PostgreSQL database, and you will see both genre
and book
tables.
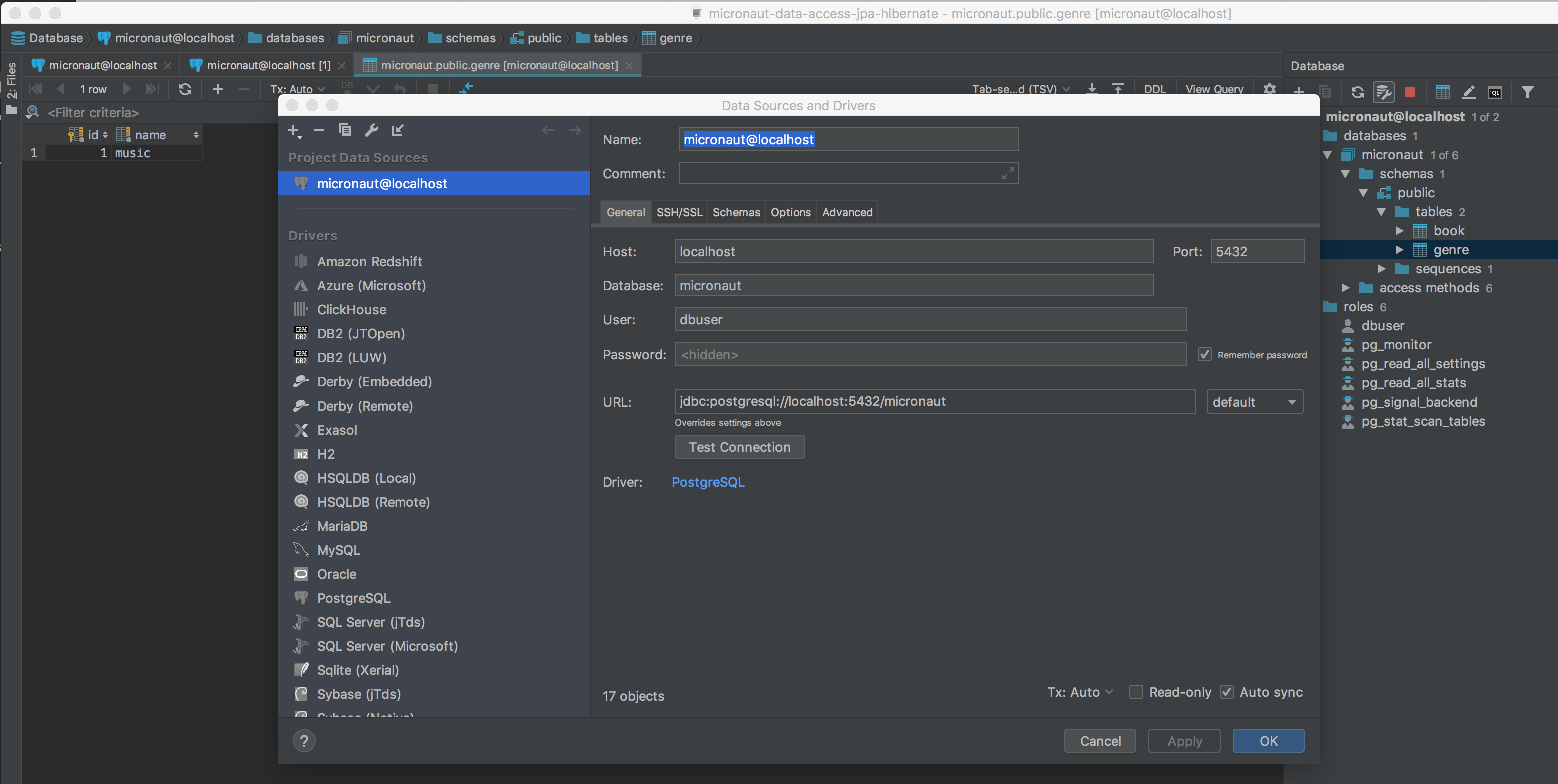
Save one genre, and your genre
table will now contain an entry.
curl -X "POST" "http://localhost:8080/genres" \
-H 'Content-Type: application/json; charset=utf-8' \
-d $'{ "name": "music" }'
8. Next Steps
Read more about Configurations for Data Access section in the Micronaut documentation.
9. Help with the Micronaut Framework
The Micronaut Foundation sponsored the creation of this Guide. A variety of consulting and support services are available.
10. License
All guides are released with an Apache license 2.0 license for the code and a Creative Commons Attribution 4.0 license for the writing and media (images…). |