Client Credentials Flow with Micronaut and Auth0
Learn how to use Client Credentials Flow between Micronaut microservices with an Authorization Server provided by Auth0.
Authors: Sergio del Amo
Micronaut Version: 4.9.1
1. Getting Started
In this guide, we will create a Micronaut application written in Groovy.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE (e.g. IntelliJ IDEA)
-
JDK 21 or greater installed with
JAVA_HOME
configured appropriately -
An Auth0 account.
3. Solution
We recommend that you follow the instructions in the next sections and create the application step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Application Diagram
Download the complete solution of the Consul and Micronaut Framework - Microservices Service Discovery guide. You will use the sample app as a starting point. The application contains three microservices:
-
bookcatalogue
- This returns a list of books. It uses a domain consisting of a book name and an ISBN. -
bookinventory
- This exposes an endpoint to check whether a book has sufficient stock to fulfil an order. It uses a domain consisting of a stock level and an ISBN. -
bookrecommendation
- This consumes previous services and exposes an endpoint that recommends book names that are in stock.
The bookcatalogue
service consumes endpoints exposed by the other services. The following image illustrates the original application flow:
A request to bookrecommendation
(http://localhost:8080/books
) triggers several requests through our microservices mesh.
In this guide, you are going to secure the communication between the microservices. You will use a client credentials flow and obtain an access token from an Auth0
authorization server.
5. OAuth 2.0
To provide authentication, sign in to your Auth0 account.
5.1. Create an application
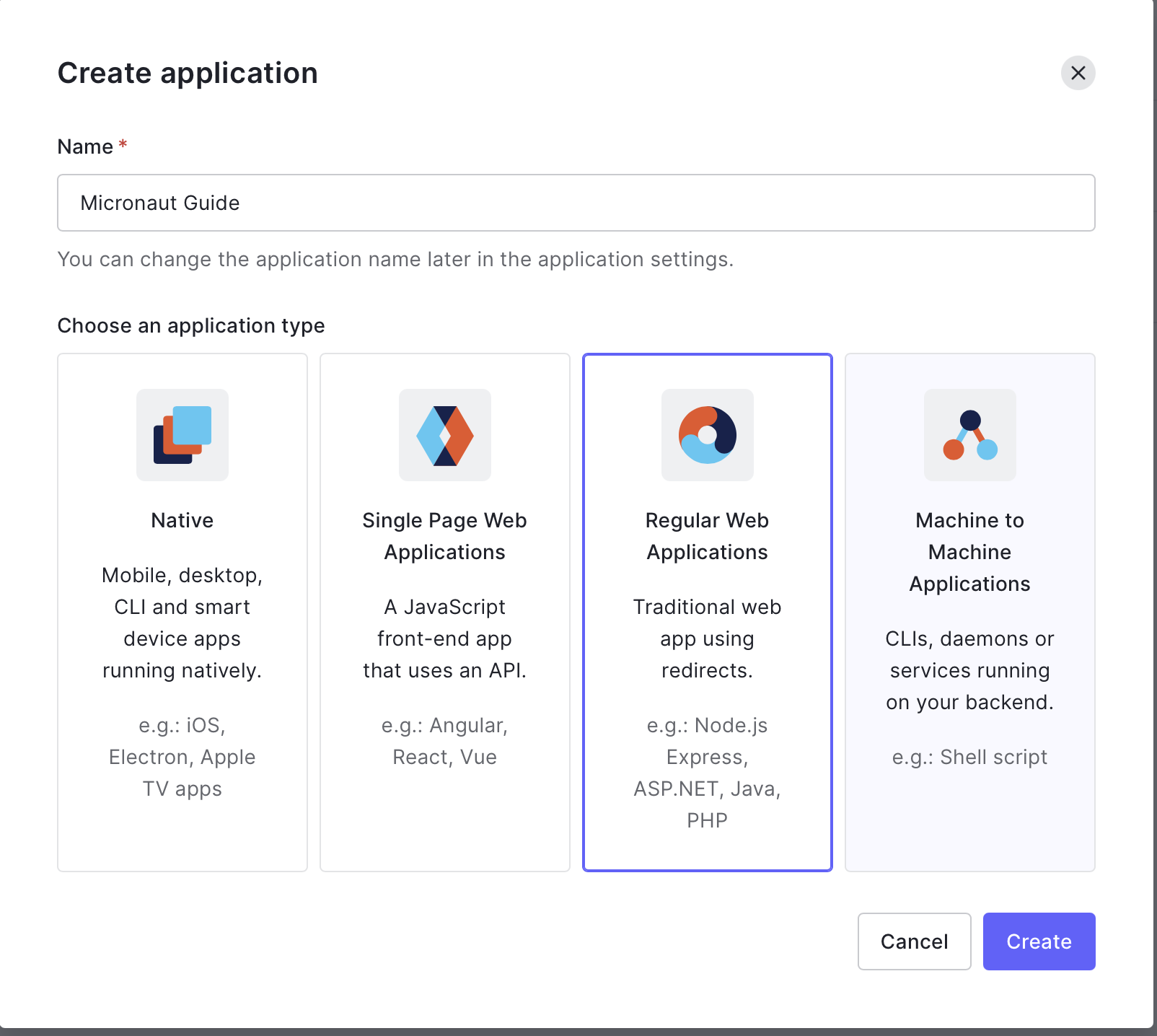
5.2. Obtain client id and client secret
You can obtain the application’s domain, client id, and secret in the Auth0 console.
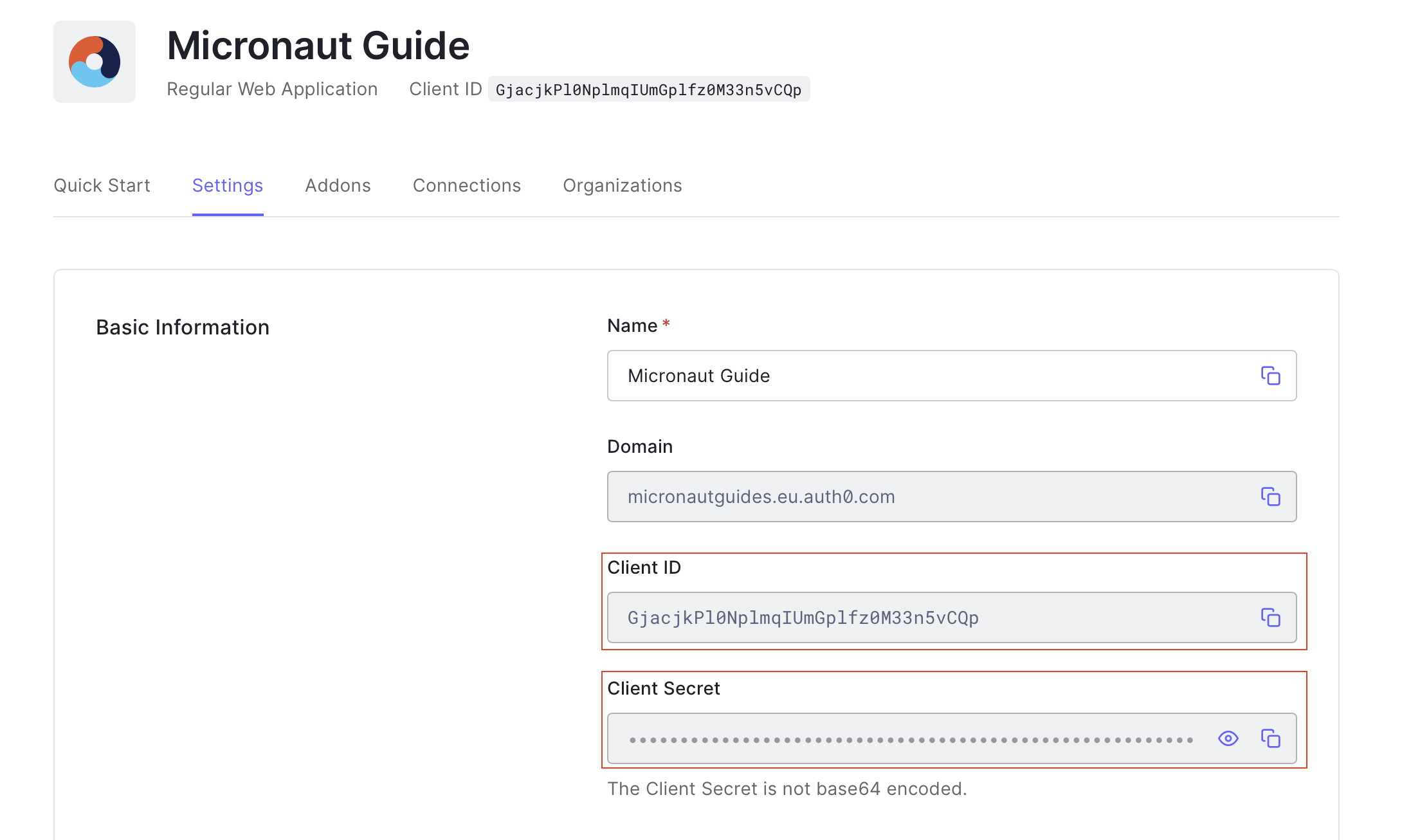
5.3. Obtain API audience
Go to Applications → APIs
and copy the API Audience:
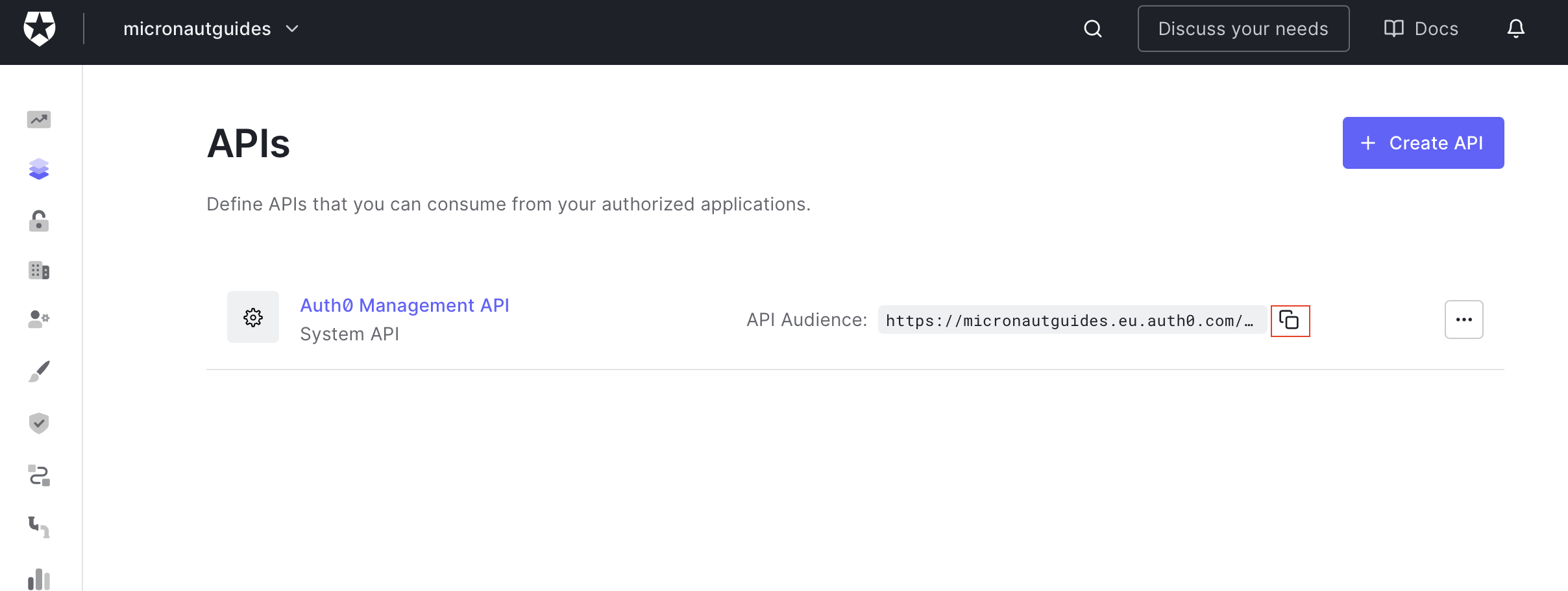
5.4. Authorize application
In the API Settings, authorize the application in the Machine to Machine Applications tab:
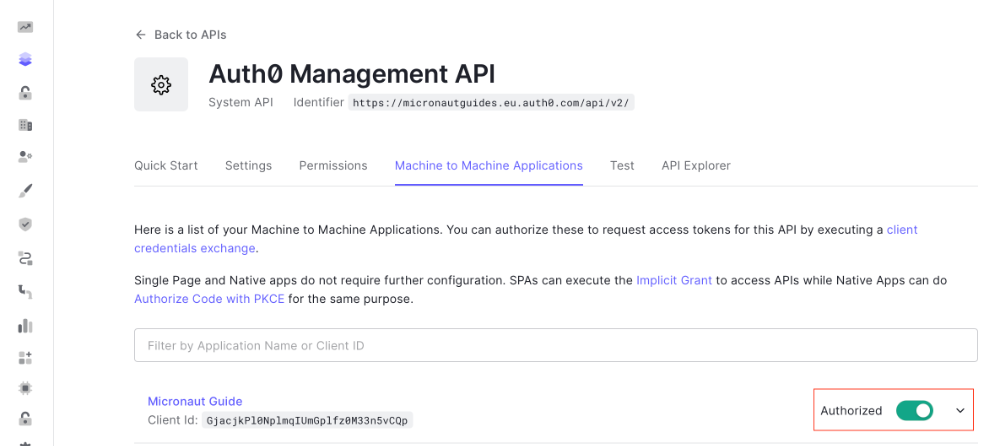
6. Writing the application
6.1. Dependencies
Modify every application (bookinventory
, bookinventory
, and bookrecommendation
). Add Micronaut JWT and Micronaut OAuth 2.0 dependencies:
<dependency>
<groupId>io.micronaut.security</groupId>
<artifactId>micronaut-security-oauth2</artifactId>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>io.micronaut.security</groupId>
<artifactId>micronaut-security-jwt</artifactId>
<scope>compile</scope>
</dependency>
6.2. Changes to Book Inventory service
Annotate the Controller’s method with @Secured
:
package example.micronaut
import groovy.transform.CompileStatic
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import io.micronaut.http.annotation.Produces
import jakarta.validation.constraints.NotBlank
import static io.micronaut.http.MediaType.TEXT_PLAIN
import static io.micronaut.security.rules.SecurityRule.IS_AUTHENTICATED;
import io.micronaut.security.annotation.Secured
@CompileStatic
@Controller("/books")
class BooksController {
@Produces(TEXT_PLAIN)
@Get("/stock/{isbn}")
@Secured(IS_AUTHENTICATED) (1)
Boolean stock(@NotBlank String isbn) {
bookInventoryByIsbn(isbn).map(bi -> bi.stock > 0).orElse(null)
}
private Optional<BookInventory> bookInventoryByIsbn(String isbn) {
if (isbn == "1491950358") {
return Optional.of(new BookInventory(isbn, 4))
}
if (isbn == "1680502395") {
return Optional.of(new BookInventory(isbn, 0))
}
Optional.empty()
}
}
1 | Annotate with io.micronaut.security.Secured to configure secured access. The SecurityRule.IS_AUTHENTICATED expression allows only access to authenticated users. |
To validate the tokens issued by Auth0, configure Validation with Remote JWKS:
micronaut:
security:
token:
jwt:
signatures:
jwks:
auth0:
url: '${OAUTH_JWKS:`https://micronautguides.eu.auth0.com/.well-known/jwks.json`}'
You can obtain the JWKS URL in the .well-known/openid-configuration
endpoint.
6.3. Changes to Book Catalogue service
Annotate the Controller’s method with @Secured
:
package example.micronaut
import groovy.transform.CompileStatic
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import io.micronaut.security.annotation.Secured
import static io.micronaut.security.rules.SecurityRule.IS_AUTHENTICATED
@CompileStatic
@Controller("/books")
class BooksController {
@Get
@Secured(IS_AUTHENTICATED) (1)
List<Book> index() {
[
new Book("1491950358", "Building Microservices"),
new Book("1680502395", "Release It!"),
new Book("0321601912", "Continuous Delivery:")
]
}
}
1 | Annotate with io.micronaut.security.Secured to configure secured access. The SecurityRule.IS_AUTHENTICATED expression allows only access to authenticated users. |
To validate the tokens issued by Auth0, configure Validation with Remote JWKS:
micronaut:
security:
token:
jwt:
signatures:
jwks:
auth0:
url: '${OAUTH_JWKS:`https://micronautguides.eu.auth0.com/.well-known/jwks.json`}'
You can obtain the JWKS URL in the .well-known/openid-configuration
endpoint.
6.4. Changes to Book Recommendations service
6.4.1. Books Controller Security
The GET /books
in the booksrecommendation
service is open.
Annotate the Controller’s method with @Secured
:
package example.micronaut
import groovy.transform.CompileStatic
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import org.reactivestreams.Publisher
import reactor.core.publisher.Flux
import static io.micronaut.security.rules.SecurityRule.IS_ANONYMOUS
import io.micronaut.security.annotation.Secured
@CompileStatic
@Controller("/books")
class BookController {
private final BookCatalogueOperations bookCatalogueOperations
private final BookInventoryOperations bookInventoryOperations
BookController(BookCatalogueOperations bookCatalogueOperations,
BookInventoryOperations bookInventoryOperations) {
this.bookCatalogueOperations = bookCatalogueOperations
this.bookInventoryOperations = bookInventoryOperations
}
@Get
@Secured(IS_ANONYMOUS) (1)
Publisher<BookRecommendation> index() {
Flux.from(bookCatalogueOperations.findAll())
.flatMap(b -> Flux.from(bookInventoryOperations.stock(b.isbn))
.filter(Boolean::booleanValue)
.map(rsp -> b)
).map(book -> new BookRecommendation(book.name))
}
}
1 | Annotate with io.micronaut.security.Secured to configure secured access. The SecurityRule.IS_ANONYMOUS expression will allow access without authentication. |
6.4.2. Configuration of HTTP Services URLs
Modify application-dev.yml
to point the declarative HTTP clients to the other microservices URLs.
micronaut:
http:
services:
bookcatalogue:
url: 'http://localhost:8081'
bookinventory:
url: 'http://localhost:8082'
6.5. Configuration
Add the following OAuth2 configuration:
micronaut:
security:
oauth2:
clients:
auth0: (1)
client-id: '${OAUTH_CLIENT_ID:xxx}' (2)
client-secret: '${OAUTH_CLIENT_SECRET:yyy}' (3)
grant-type: 'client_credentials' (4)
token:
url: '${OAUTH_TOKEN_URL:`https://micronautguides.eu.auth0.com/oauth/token`}' (5)
auth-method: 'client_secret_post' (6)
client-credentials:
service-id-regex: 'bookcatalogue|bookinventory' (7)
additional-request-params:
audience: '${AUTH0_API_IDENTIFIER:`https://micronautguides.eu.auth0.com/api/v2/`}' (8)
1 | OAuth 2.0 client name. |
2 | Client id. See previous screenshot. |
3 | Client secret. See previous screenshot. |
4 | Specify GrantType#CLIENT_CREDENTIALS client-credentials as grant type for this OAuth 2.0 client. |
5 | Specify the token endpoint URL. You can obtain the token endpoint URL in the .well-known/openid-configuration . |
6 | Specify AuthenticationMethod#CLIENT_SECRET_POST as the authentication method. This means the client id and client secret are specified in the body of the HTTP request sent to the token endpoint. |
7 | Propagate the access token obtained from Auth0 to requests sent to the services bookinventory and bookcatalogue . This uses the Micronaut Client Credentials HTTP Client Filter. |
8 | Auth0 requires the API Identifier with an audience key in the token endpoint request for the client credentials flow. |
The previous configuration uses several placeholders with default values. You will need to set up OAUTH_CLIENT_ID
, OAUTH_CLIENT_SECRET
, and OAUTH_TOKEN_URL
environment variables in your Auth0 application.
export OAUTH_CLIENT_ID=XXXXXXXXXX
export OAUTH_CLIENT_SECRET=YYYYYYYYYY
export OAUTH_TOKEN_URL=https://micronautguides.eu.auth0.com/oauth/token
7. Running the Application
7.1. Run bookcatalogue
microservice
To run the application, execute ./mvnw mn:run
.
...
14:28:34.034 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 499ms. Server Running: http://localhost:8081
7.2. Run bookinventory
microservice
To run the application, execute ./mvnw mn:run
.
...
14:31:13.104 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 506ms. Server Running: http://localhost:8082
7.3. Run bookrecommendation
microservice
To run the application, execute ./mvnw mn:run
.
...
14:31:57.389 [main] INFO io.micronaut.runtime.Micronaut - Startup completed in 523ms. Server Running: http://localhost:8080
You can run a cURL command to test the whole application:
curl http://localhost:8080/books
[{"name":"Building Microservices"}]
8. Next Steps
Read Micronaut OAuth 2.0 Documentation to learn more.
9. Help with the Micronaut Framework
The Micronaut Foundation sponsored the creation of this Guide. A variety of consulting and support services are available.
10. License
All guides are released with an Apache license 2.0 license for the code and a Creative Commons Attribution 4.0 license for the writing and media (images…). |