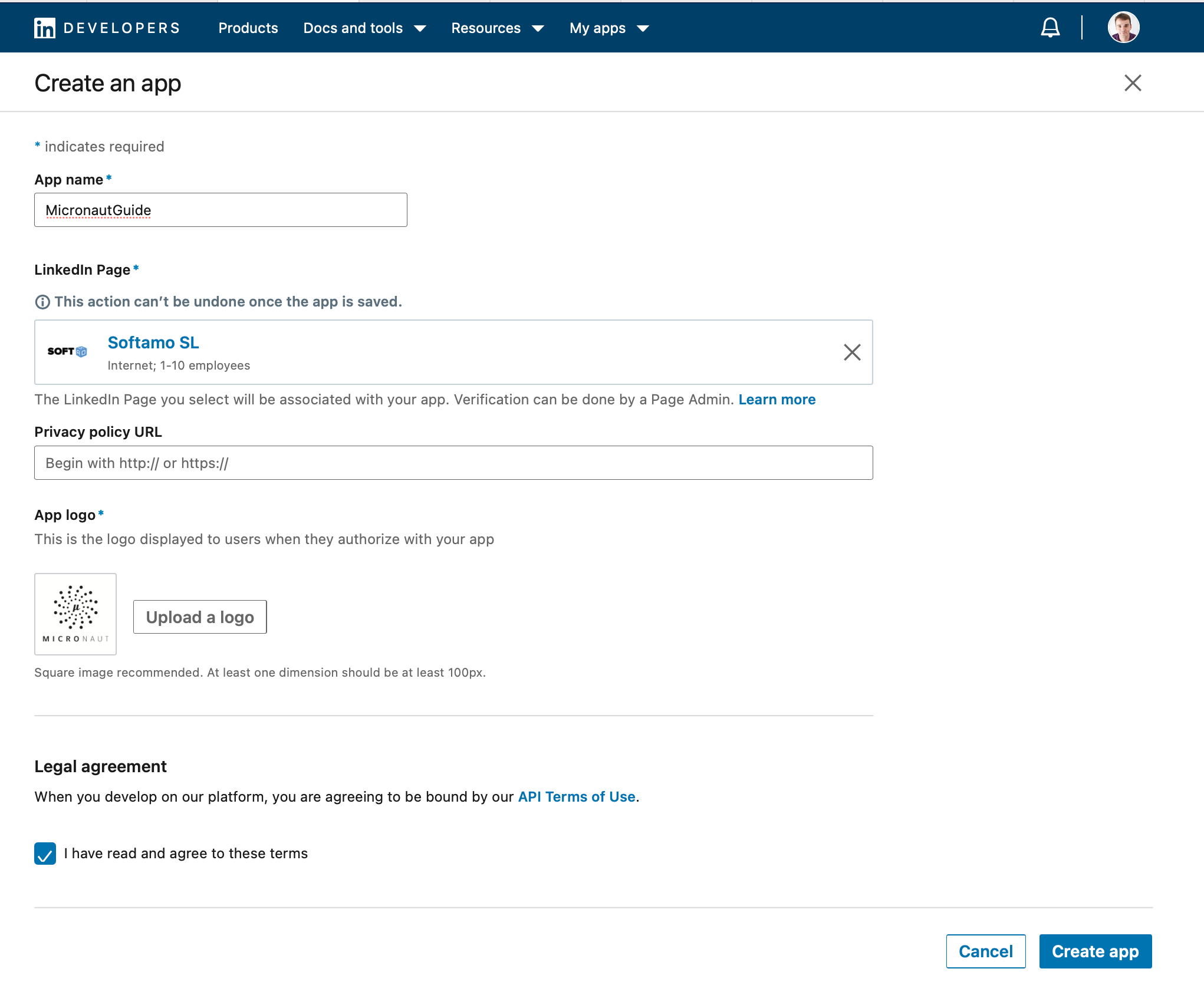
Secure a Micronaut application with LinkedIn
Learn how to create a Micronaut application and authenticate with LinkedIn.
Authors: Sergio del Amo
Micronaut Version: 4.9.0
1. Getting Started
In this guide, we will create a Micronaut application written in Groovy.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE (e.g. IntelliJ IDEA)
-
JDK 21 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the application step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. OAuth 2.0
To provide Sign in with LinkedIn with Authorization Code Flow, you need to visit linkedin.com/developers/ and:
4.1. Create a LinkedIn App
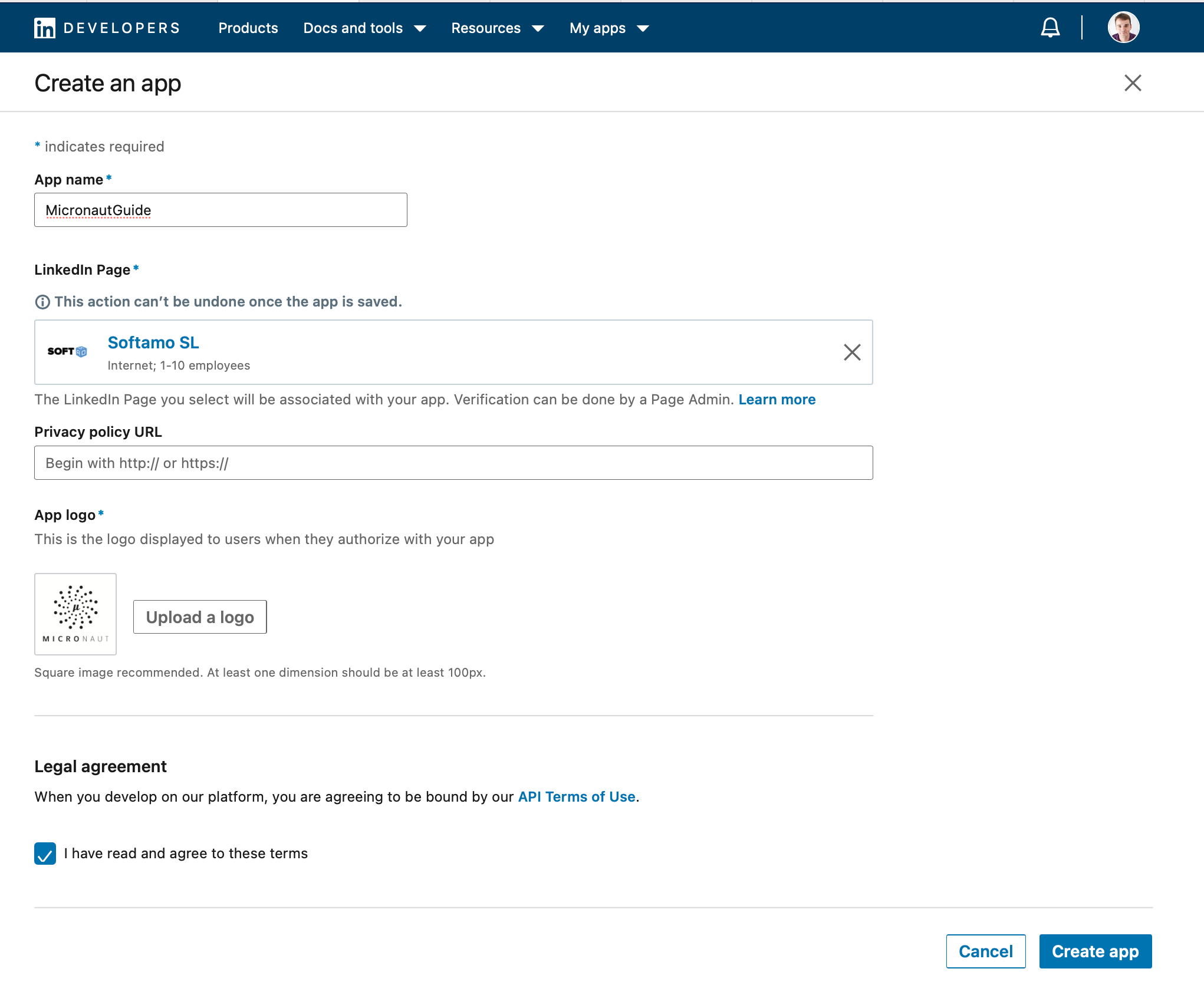
4.2. Add Sign in with LinkedIn Product
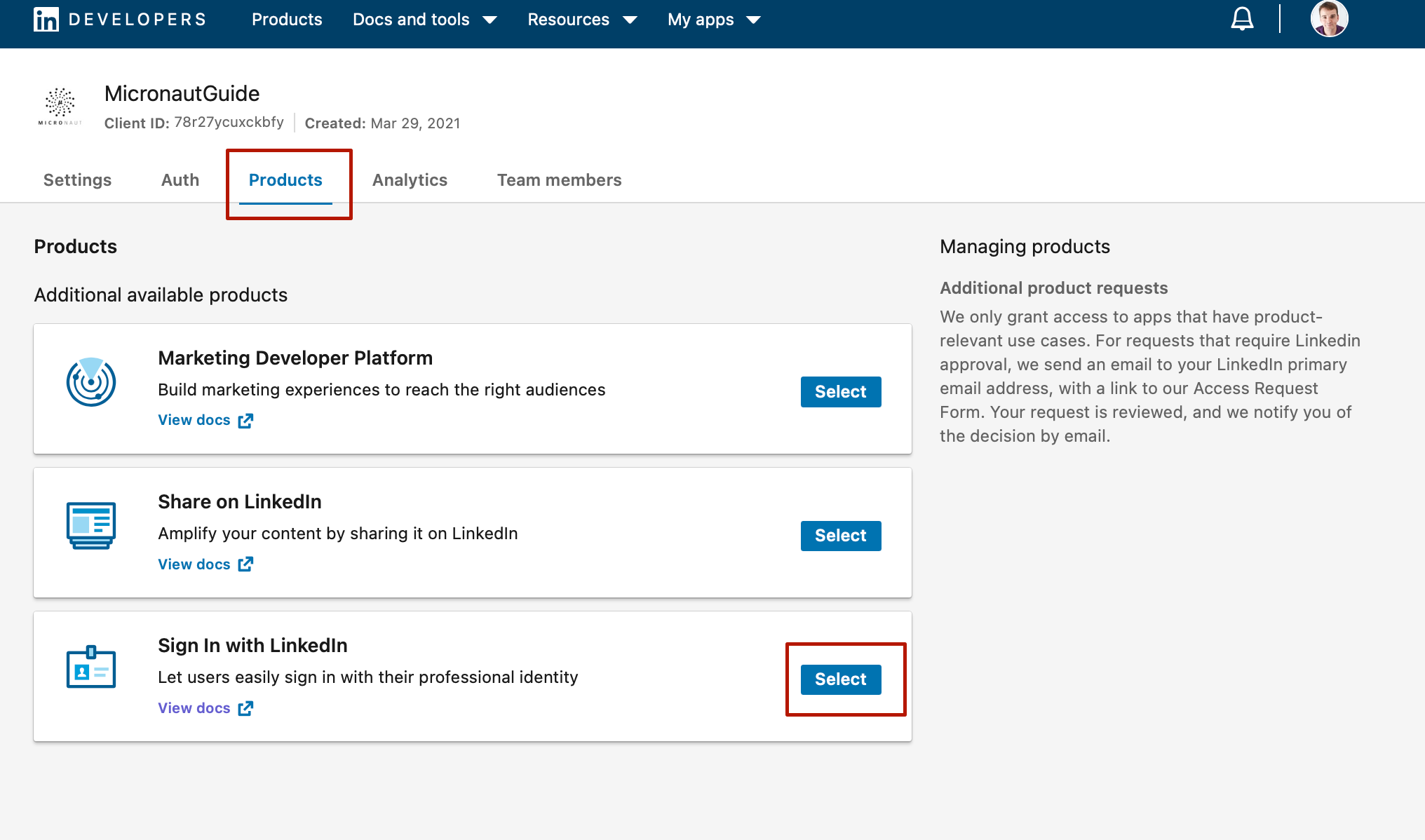
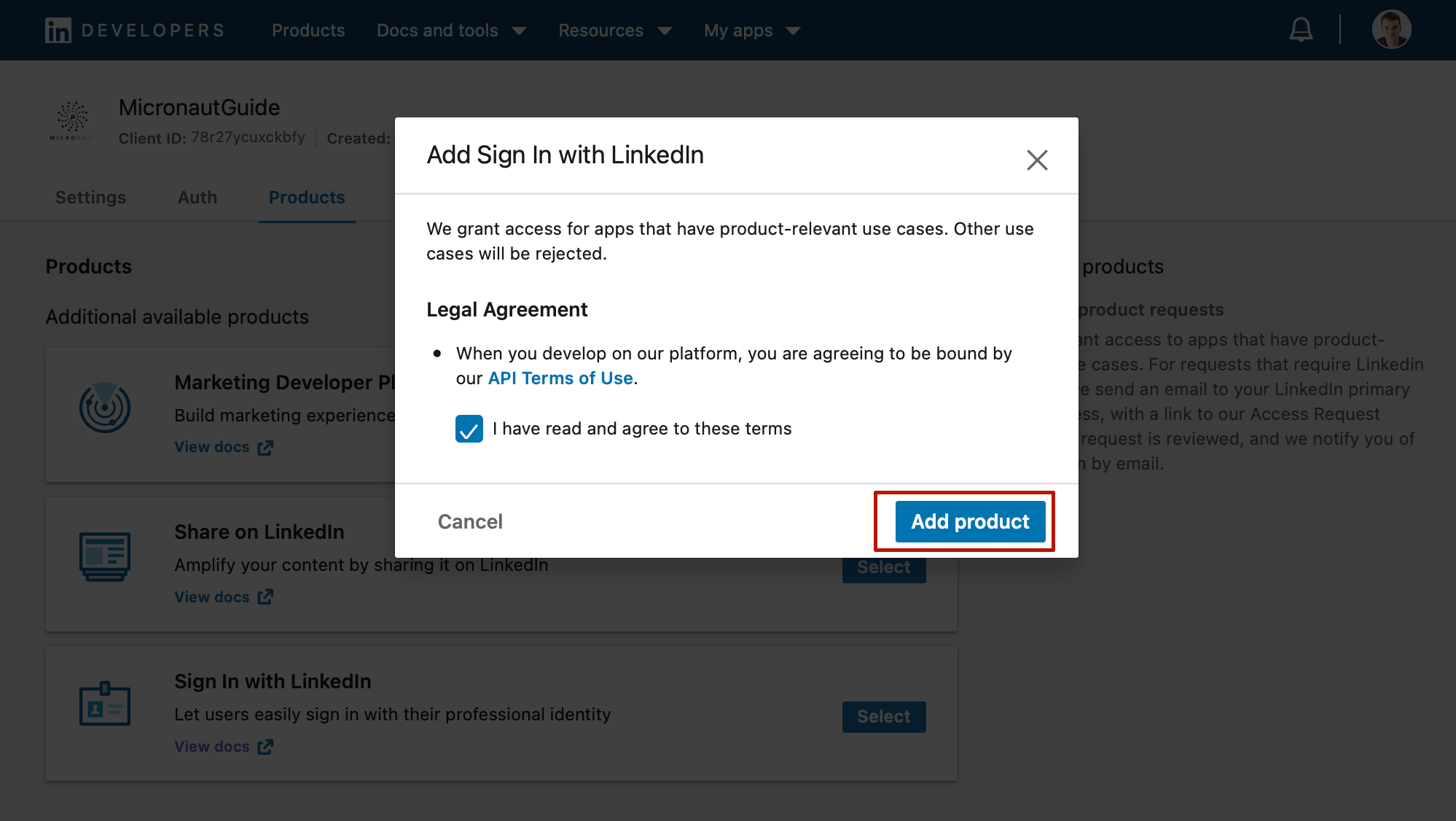
4.3. Configure Authorized Redirect URL
Go to the Auth
tab.
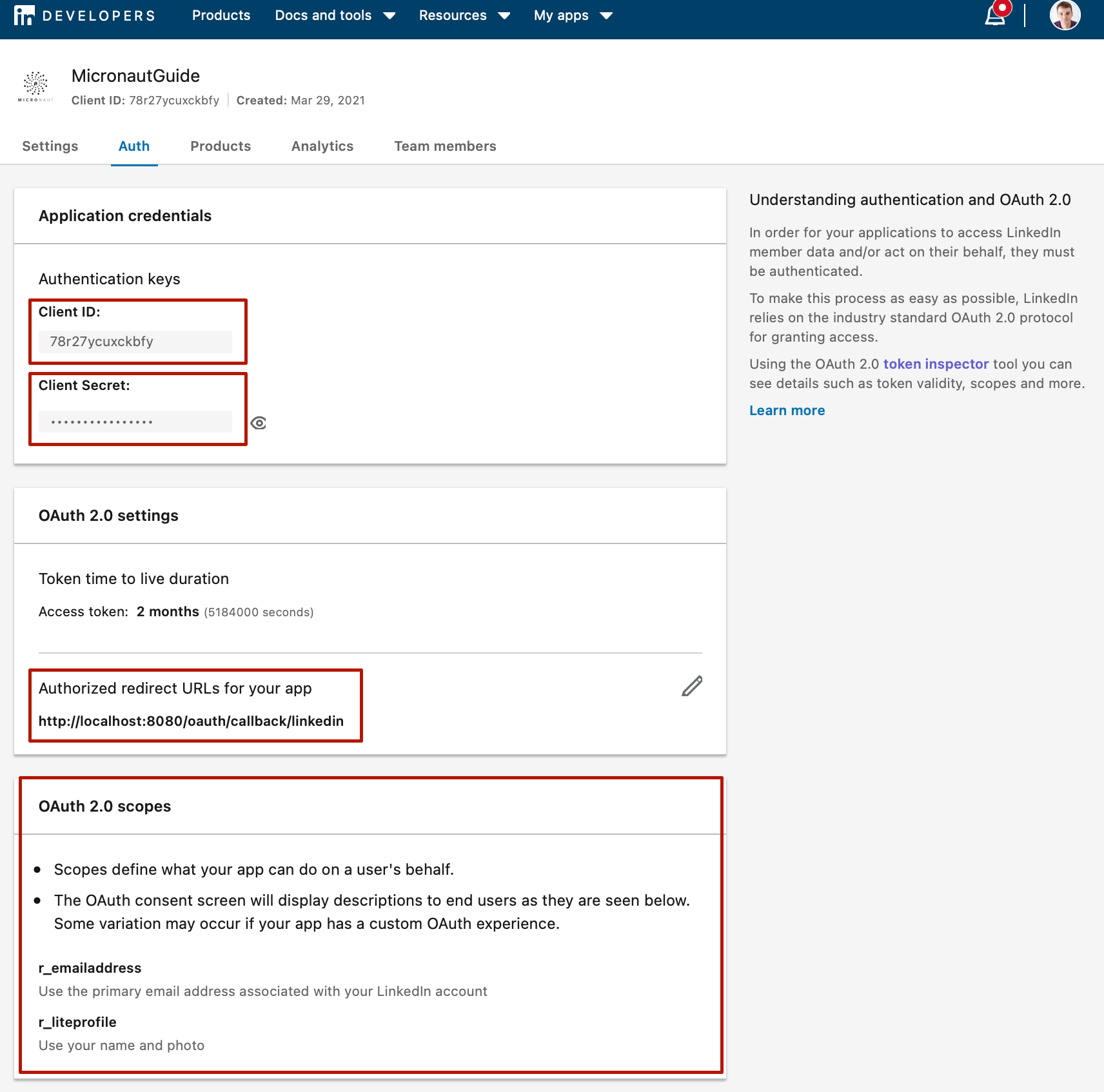
-
Save your client id and client secret. You will need them to configure your Micronaut applications.
-
Register
http://localhost:8080/oauth/callback/linkedin
. By creating a client namedlinkedin
in the Micronaut application, route/oauth/callback/linkedin
is registered. -
After you add
Add Sign in with LinkedIn
the scopesr_liteprofile
andr_emailaddress
will appear. We will configure the Micronaut application to request scoper_liteprofile
.
5. Writing the Application
Create an application using the Micronaut Command Line Interface or with Micronaut Launch.
mn create-app example.micronaut.micronautguide \
--features=yaml,security-oauth2,security-jwt,views-thymeleaf,reactor,serialization-jackson,http-client \
--build=maven \
--lang=groovy \
--test=spock
If you don’t specify the --build argument, Gradle with the Kotlin DSL is used as the build tool. If you don’t specify the --lang argument, Java is used as the language.If you don’t specify the --test argument, JUnit is used for Java and Kotlin, and Spock is used for Groovy.
|
The previous command creates a Micronaut application with the default package example.micronaut
in a directory named micronautguide
.
If you use Micronaut Launch, select Micronaut Application as application type and add yaml
, security-oauth2
, security-jwt
, views-thymeleaf
, reactor
, serialization-jackson
, and http-client
features.
If you have an existing Micronaut application and want to add the functionality described here, you can view the dependency and configuration changes from the specified features, and apply those changes to your application. |
5.1. Views
Although the Micronaut framework is primarily designed around message encoding / decoding, there are occasions where it is convenient to render a view on the server side.
We’ll use the Thymeleaf Java template engine to render views.
5.2. Home
Create a controller to handle the requests to /
. You will display the email of the authenticated person if any. Annotate the controller endpoint with @View
since we will use a Thymeleaf template.
package example.micronaut
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import io.micronaut.security.annotation.Secured
import io.micronaut.security.rules.SecurityRule
import io.micronaut.views.View
@Controller (1)
class HomeController {
@Secured(SecurityRule.IS_ANONYMOUS) (2)
@View('home') (3)
@Get (4)
Map<String, Object> index() {
[:]
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path / . |
2 | Annotate with io.micronaut.security.Secured to configure secured access. The SecurityRule.IS_ANONYMOUS expression will allow access without authentication. |
3 | Use View annotation to specify which template to use to render the response. |
4 | The @Get annotation maps the index method to GET / requests. |
Create a Thymeleaf template:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Home</title>
</head>
<body>
<h1>Micronaut - LinkedIn example</h1>
<h2 th:if="${security}">username: <span th:text="${security.name}"></span></h2>
<h2 th:unless="${security}">username: Anonymous</h2>
<nav>
<ul>
<li th:unless="${security}"><a href="/oauth/login/linkedin">Enter</a></li>
<li th:if="${security}"><a href="/logout">Logout</a></li>
</ul>
</nav>
</body>
</html>
Also, note that we return an empty model in the controller. However, we are accessing security
in the Thymeleaf template.
-
The SecurityViewModelProcessor injects into the model a
security
map with the authenticated user. See User in a view documentation.
5.3. OAuth 2.0 authorization code grant flow
Here is a high level diagram of how the authorization code grant flow works with an OAuth 2.0 provider such as LinkedIn.
5.4. OAuth 2.0 Configuration
Add the following OAuth2 Configuration:
micronaut:
security:
authentication: cookie (1)
token:
jwt:
signatures:
secret:
generator: (2)
secret: '${JWT_GENERATOR_SIGNATURE_SECRET:pleaseChangeThisSecretForANewOne}' (3)
oauth2:
clients:
linkedin: (4)
client-id: '${OAUTH_CLIENT_ID:xxx}' (5)
client-secret: '${OAUTH_CLIENT_SECRET:yyy}' (6)
scopes:
- r_liteprofile (7)
authorization:
url: https://www.linkedin.com/oauth/v2/authorization (8)
token:
url: https://www.linkedin.com/oauth/v2/accessToken (9)
endpoints:
logout:
get-allowed: true (10)
1 | Set micronaut.security.authentication to cookie to generate a signed JWT once the user is authenticated. The token is saved in a Cookie and read in subsequent requests. |
2 | You can create a SecretSignatureConfiguration named generator via configuration as illustrated above. The generator signature is used to sign the issued JWT claims. |
3 | Change this value to your own secret and keep it safe (do not store this in your VCS). |
4 | The provider identifier must match the last part of the URL you entered as a redirect URL: /oauth/callback/linkedin . |
5 | Client Secret. See previous screenshot. |
6 | Client ID. See previous screenshot. |
7 | Specify the scopes you want to request. Linked Permission Types let you specify exactly what type of access you need. |
8 | Map manually the LinkedIn’s Authorization endpoint. |
9 | Map manually the LinkedIn’s Token endpoint. |
10 | Accept GET request to the /logout endpoint. |
The previous configuration uses several placeholders. You will need to set up OAUTH_CLIENT_ID
, OAUTH_CLIENT_SECRET
environment variables.
export OAUTH_CLIENT_ID=XXXXXXXXXX export OAUTH_CLIENT_SECRET=YYYYYYYYYY
5.5. OAuth 2.0 Details Mapper
We need an HTTP Client to retrieve the user info. Create a POJO to represent a LinkedIn user:
package example.micronaut
import groovy.transform.CompileStatic
import io.micronaut.core.annotation.NonNull
import io.micronaut.serde.annotation.Serdeable
import jakarta.validation.constraints.NotBlank
@CompileStatic
@Serdeable
class LinkedInMe {
@NonNull
@NotBlank
final String id
@NonNull
@NotBlank
final String localizedFirstName
@NonNull
@NotBlank
final String localizedLastName
LinkedInMe(@NonNull String id,
@NonNull String localizedFirstName,
@NonNull String localizedLastName) {
this.id = id
this.localizedFirstName = localizedFirstName
this.localizedLastName = localizedLastName
}
}
Then, create a Micronaut Declarative HTTP Client to consume LinkedIn Profile endpoint
package example.micronaut
import groovy.transform.CompileStatic
import io.micronaut.http.annotation.Get
import io.micronaut.http.annotation.Header
import io.micronaut.http.client.annotation.Client
import org.reactivestreams.Publisher
@CompileStatic
@Client(id = 'linkedin') (1)
interface LinkedInApiClient {
@Get('/v2/me') (2)
Publisher<LinkedInMe> me(@Header String authorization) (3) (4)
}
1 | Add the id linkedin to the @Client annotation. Later, you will provide URL for this client id. |
2 | Define a HTTP GET request to /v2/me endpoint. |
3 | You can return reactive types in a Micronaut declarative HTTP client. |
4 | You can specify that a parameter binds to a HTTP Header such as the Authorization header. |
Specify the URL for the linkedin
service.
micronaut:
http:
services:
linkedin:
url: "https://api.linkedin.com"
Create an implementation of OauthAuthenticationMapper. The implementation must be qualified by a name that matches the name present in the client configuration. The value specified in the client configuration is linkedin
.
package example.micronaut
import groovy.transform.CompileStatic
import io.micronaut.core.annotation.Nullable
import io.micronaut.security.authentication.AuthenticationResponse
import io.micronaut.security.oauth2.endpoint.authorization.state.State
import io.micronaut.security.oauth2.endpoint.token.response.OauthAuthenticationMapper
import io.micronaut.security.oauth2.endpoint.token.response.TokenResponse
import jakarta.inject.Named
import jakarta.inject.Singleton
import org.reactivestreams.Publisher
import reactor.core.publisher.Mono
import static io.micronaut.http.HttpHeaderValues.AUTHORIZATION_PREFIX_BEARER
@CompileStatic
@Named('linkedin') (1)
@Singleton (2)
class LinkedInOauthAuthenticationMapper implements OauthAuthenticationMapper {
private final LinkedInApiClient linkedInApiClient
LinkedInOauthAuthenticationMapper(LinkedInApiClient linkedInApiClient) { (3)
this.linkedInApiClient = linkedInApiClient
}
@Override
Publisher<AuthenticationResponse> createAuthenticationResponse(TokenResponse tokenResponse,
@Nullable State state) {
Mono.from(linkedInApiClient.me(AUTHORIZATION_PREFIX_BEARER + ' ' + tokenResponse.accessToken))
.map(linkedMe -> {
Map<String, Object> attributes = [
'firstName': linkedMe.localizedFirstName,
'lastName': linkedMe.localizedLastName] as Map
String username = linkedMe.id
return AuthenticationResponse.success(username, Collections.emptyList(), attributes)
})
}
}
1 | Declare @Named at the class level of a bean to explicitly define the name of the bean. |
2 | Use jakarta.inject.Singleton to designate a class as a singleton. |
3 | Use constructor injection to inject a bean of type LinkedInApiClient . |
6. Running the Application
To run the application, use the ./mvnw mn:run
command, which starts the application on port 8080.
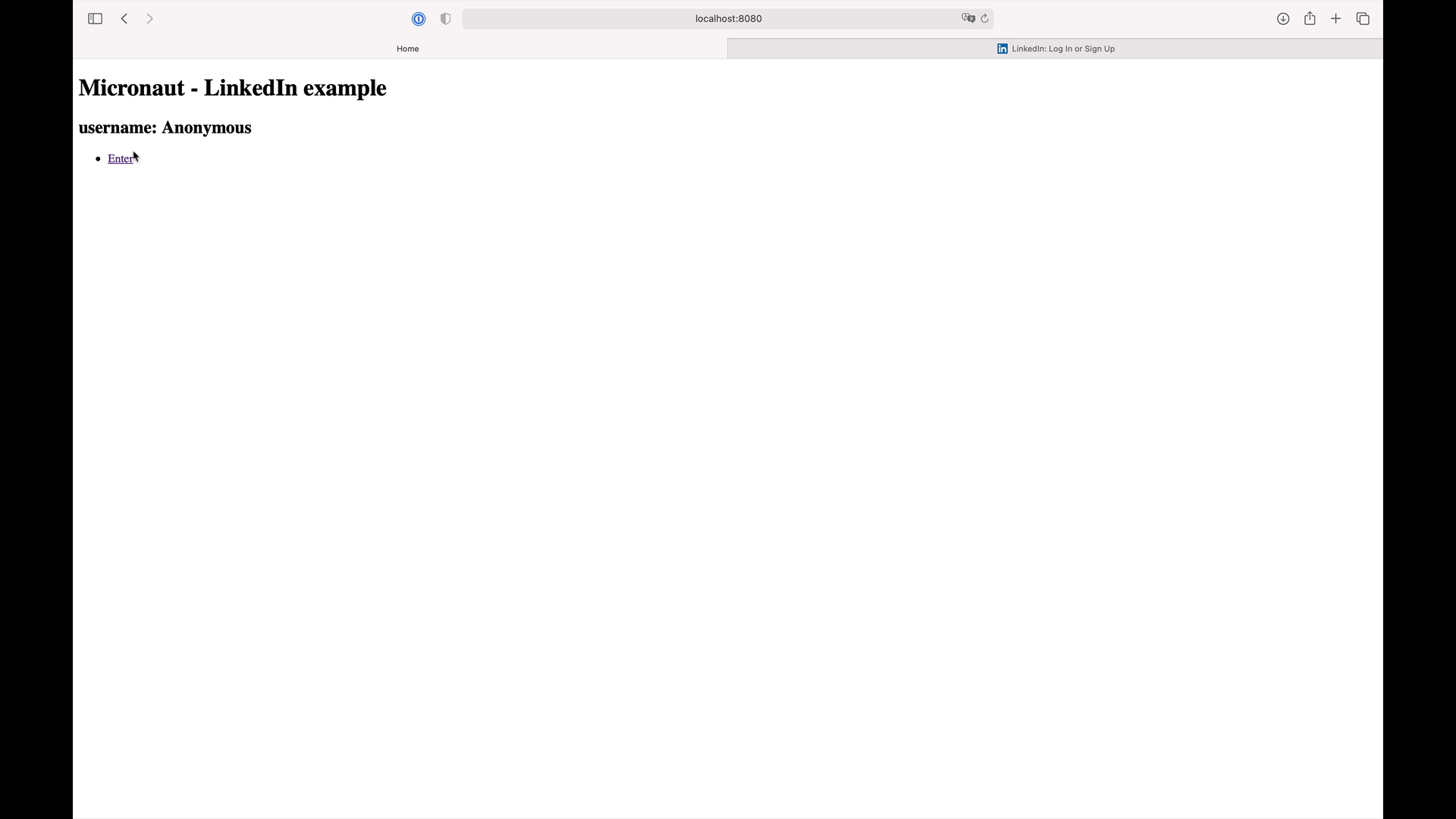
7. Next Steps
Read Micronaut OAuth 2.0 documentation to learn more.
8. Help with the Micronaut Framework
The Micronaut Foundation sponsored the creation of this Guide. A variety of consulting and support services are available.
9. License
All guides are released with an Apache license 2.0 license for the code and a Creative Commons Attribution 4.0 license for the writing and media (images…). |