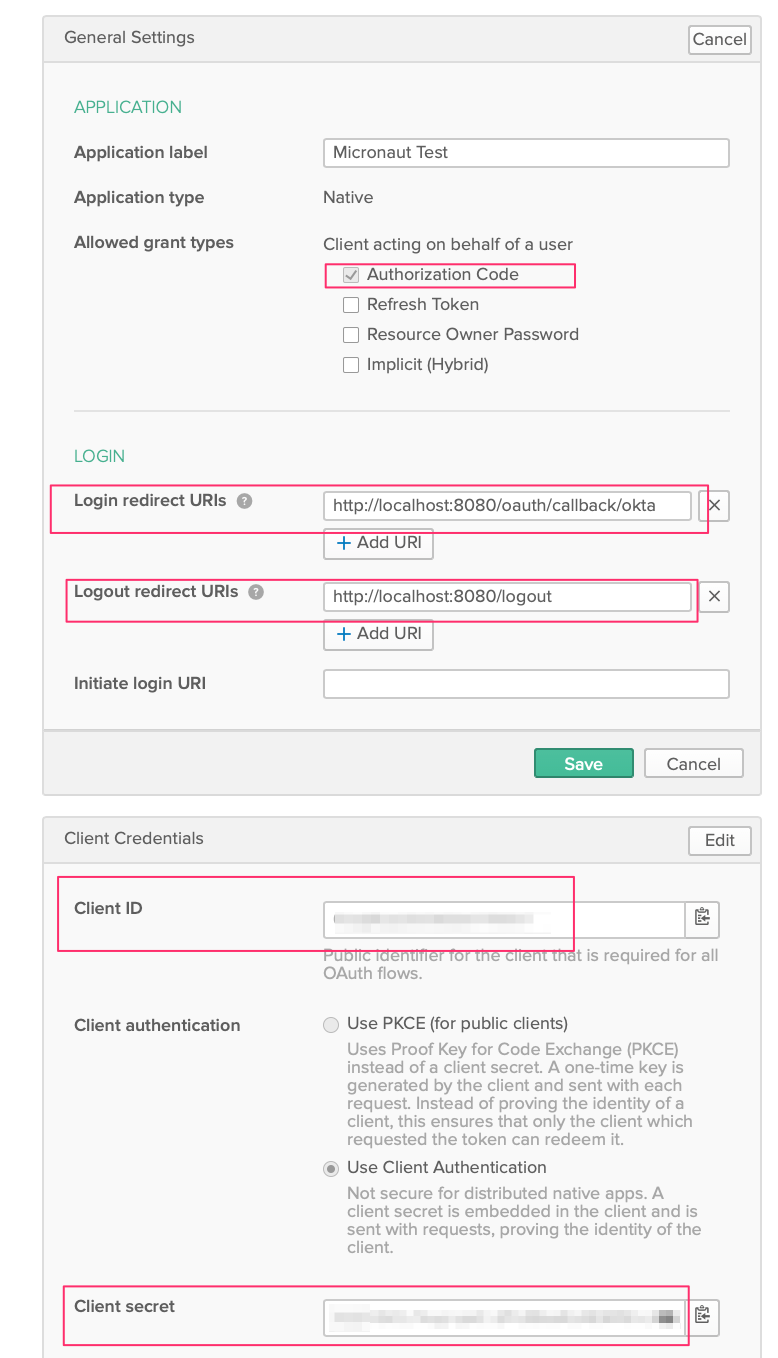
Secure a Micronaut application with Okta
Learn how to create a Micronaut application and secure it with an Authorization Server provided by Okta.
Authors: Sergio del Amo
Micronaut Version: 4.6.3
1. Getting Started
In this guide, we will create a Micronaut application written in Java.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE (e.g. IntelliJ IDEA)
-
JDK 21 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the application step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. OAuth 2.0
Sign up at developer.okta.com and create a Web application with the following characteristics:
-
Check
Authorization Code
grant type. -
Add
http://localhost:8080/oauth/callback/okta
as a login redirect URIs. -
Add
http://localhost:8080/logout
as a Logout redirect URI. -
Annotate the Client ID and Secret.
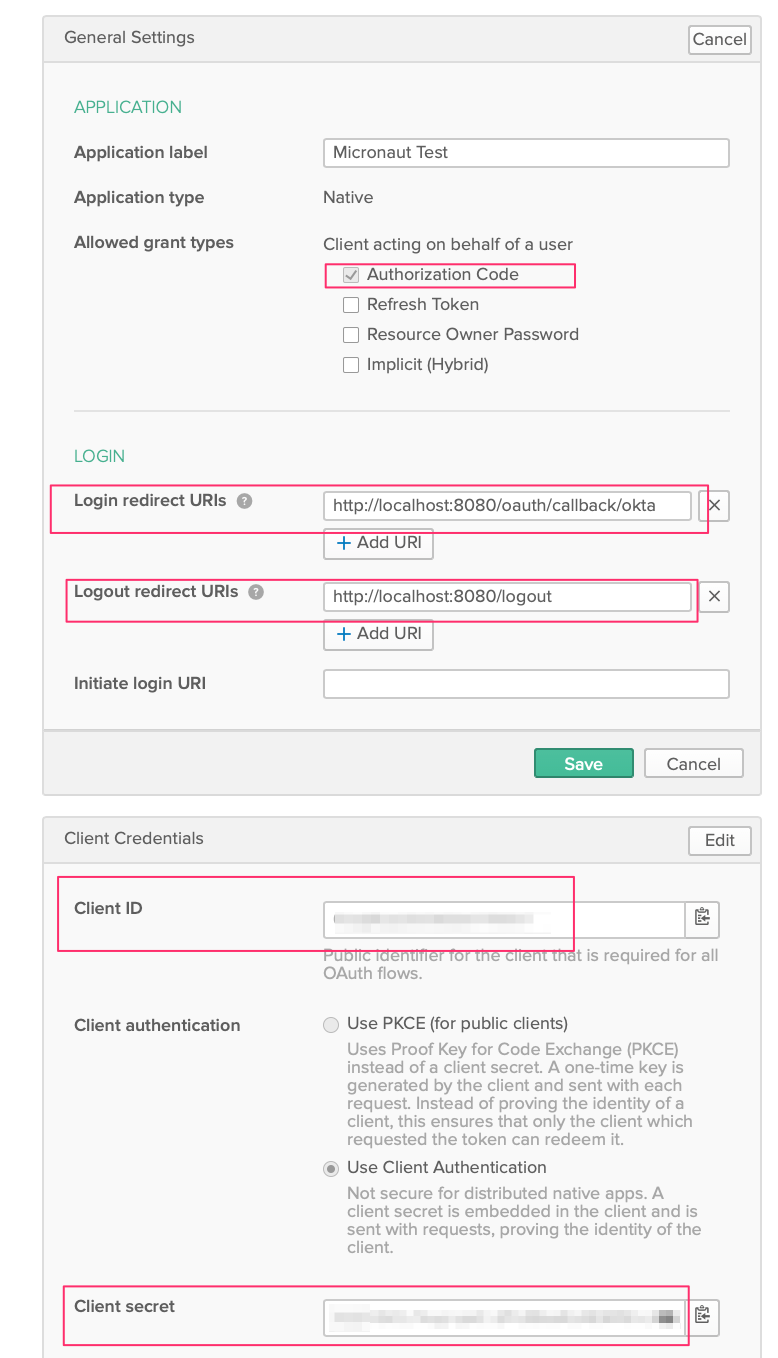
5. Writing the Application
Create an application using the Micronaut Command Line Interface or with Micronaut Launch.
mn create-app example.micronaut.micronautguide --build=gradle --lang=java
If you don’t specify the --build argument, Gradle with the Kotlin DSL is used as the build tool. If you don’t specify the --lang argument, Java is used as the language.If you don’t specify the --test argument, JUnit is used for Java and Kotlin, and Spock is used for Groovy.
|
The previous command creates a Micronaut application with the default package example.micronaut
in a directory named micronautguide
.
5.1. Security Dependencies
To use OAuth 2.0 integration, add the following dependency:
implementation("io.micronaut.security:micronaut-security-oauth2")
Also add JWT Micronaut JWT support dependencies:
implementation("io.micronaut.security:micronaut-security-jwt")
6. Views
To use the Thymeleaf Java template engine to render views in a Micronaut application, add the following dependency on your classpath.
implementation("io.micronaut.views:micronaut-views-thymeleaf")
6.1. Configuration
Add the following OAuth2 Configuration:
(1)
micronaut.security.authentication=idtoken
(2)
micronaut.security.oauth2.clients.okta.client-secret=${OAUTH_CLIENT_SECRET:yyy}
(3)
micronaut.security.oauth2.clients.okta.client-id=${OAUTH_CLIENT_ID:xxx}
(4)
micronaut.security.oauth2.clients.okta.openid.issuer=${OIDC_ISSUER_DOMAIN:`https://dev-XXXXX.oktapreview.com`}/oauth2/${OIDC_ISSUER_AUTHSERVERID:default}
(5)
micronaut.security.endpoints.logout.get-allowed=true
1 | Set micronaut.security.authentication as idtoken . The idtoken provided by Okta when the OAuth 2.0 Authorization code flow ends will be saved in a cookie. The id token is a signed JWT. For every request, the Micronaut framework extracts the JWT from the Cookie and validates the JWT signature with the remote Json Web Key Set exposed by Okta. JWKS is exposed by the jws-uri entry of Okta .well-known/openid-configuration . |
2 | The provider identifier should match the last part of the URL you entered as a redirect URL /oauth/callback/okta |
3 | Client ID & Client Secret. See previous screenshot. |
4 | issuer URL. It allows the Micronaut framework to discover the configuration of the OpenID Connect server. |
5 | Accept GET request to the /logout endpoint. |
6.1.1. Environment Variables
The previous configuration uses several placeholders. You will need to set up OAUTH_CLIENT_ID
, OAUTH_CLIENT_SECRET
, OIDC_ISSUER_DOMAIN
and OIDC_ISSUER_AUTHSERVERID
environment variables.
export OAUTH_CLIENT_ID=XXXXXXXXXX export OAUTH_CLIENT_SECRET=YYYYYYYYYY export OIDC_ISSUER_DOMAIN=https://dev-XXXXX.oktapreview.com export OIDC_ISSUER_AUTHSERVERID=default export OAUTH_ISSUER=https://dev-XXXXX.oktapreview.com/oauth2/default
Check OKTA .well-known/openid-configuration documentation.
6.2. Authorization Code Grant
We want to use an Authorization Code grant type flow which it is described in the following diagram:
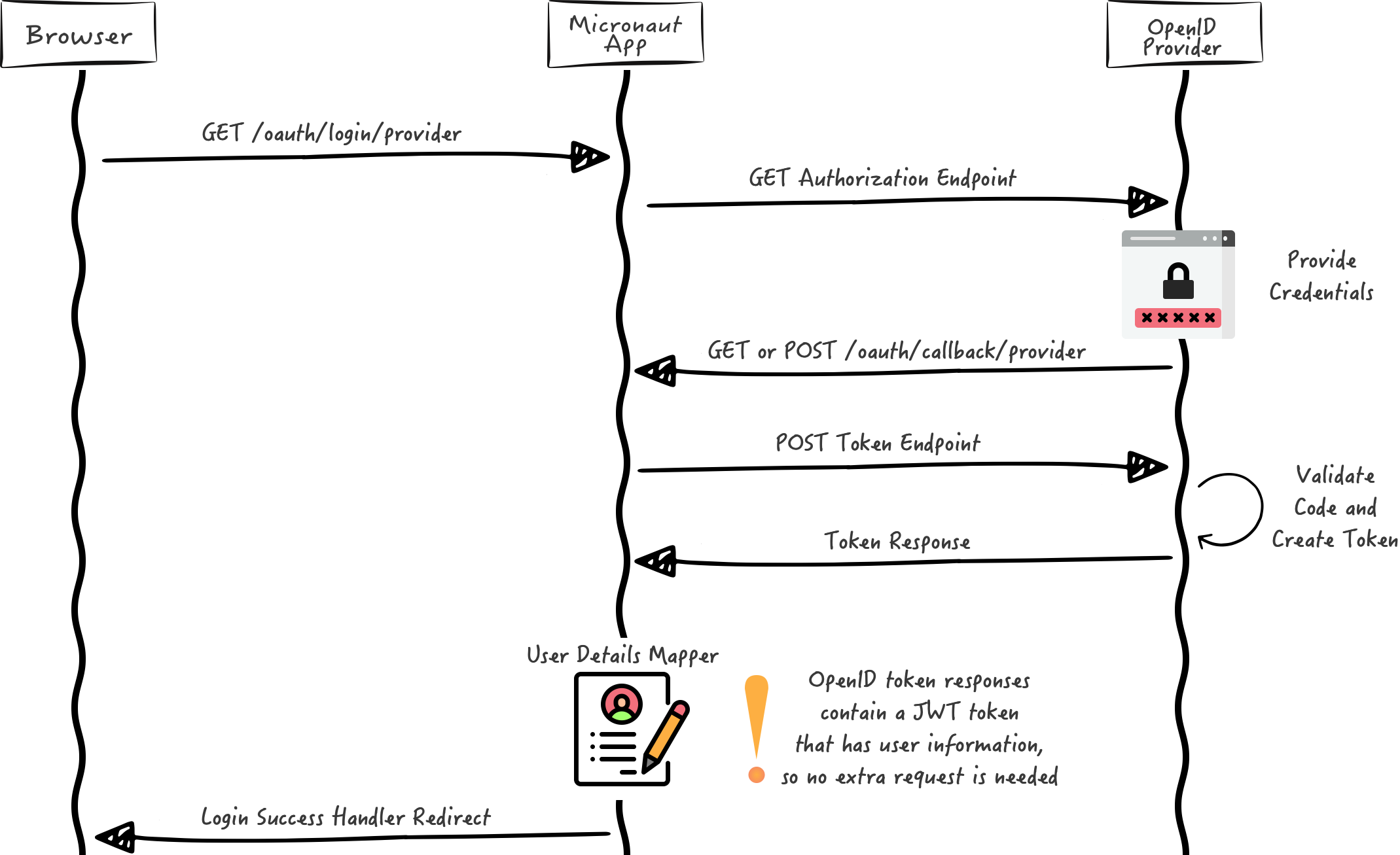
6.3. Home
Create a controller to handle the requests to /
. You will display the email of the authenticated person if any. Annotate the controller endpoint with @View
since we will use a Thymeleaf template.
package example.micronaut;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
import io.micronaut.security.annotation.Secured;
import io.micronaut.security.rules.SecurityRule;
import io.micronaut.views.View;
import java.util.HashMap;
import java.util.Map;
@Controller (1)
public class HomeController {
@Secured(SecurityRule.IS_ANONYMOUS) (2)
@View("home") (3)
@Get (4)
public Map<String, Object> index() {
return new HashMap<>();
}
}
1 | The class is defined as a controller with the @Controller annotation mapped to the path / . |
2 | Annotate with io.micronaut.security.Secured to configure secured access. The SecurityRule.IS_ANONYMOUS expression will allow access without authentication. |
3 | Use View annotation to specify which template to use to render the response. |
4 | The @Get annotation maps the index method to GET / requests. |
6.4. Thymleaf Template
Create a thymeleaf template:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Home</title>
</head>
<body>
<h1>Micronaut - Okta example</h1>
<h2 th:if="${security}">username: <span th:text="${security.attributes.get('email')}"></span></h2>
<h2 th:unless="${security}">username: Anonymous</h2>
<nav>
<ul>
<li th:unless="${security}"><a href="/oauth/login/okta">Enter</a></li>
<li th:if="${security}"><a href="/oauth/logout">Logout</a></li>
</ul>
</nav>
</body>
</html>
Also, note that we return an empty model in the controller. However, we are accessing security
in the thymeleaf template.
-
The SecurityViewModelProcessor injects into the model a
security
map with the authenticated user. See User in a view documentation.
7. Running the Application
To run the application, use the ./gradlew run
command, which starts the application on port 8080.
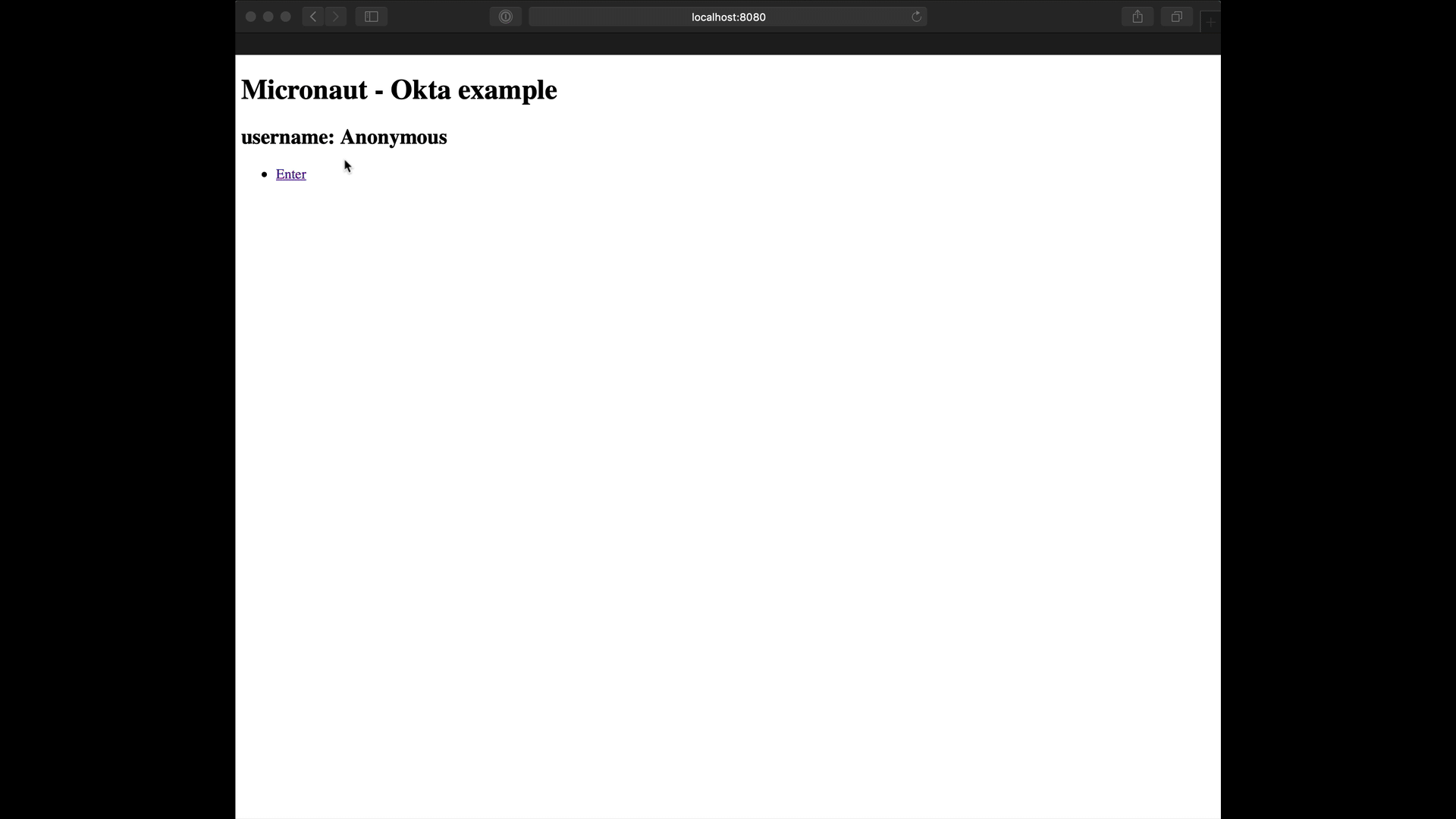
8. Generate a Micronaut Application Native Executable with GraalVM
We will use GraalVM, an advanced JDK with ahead-of-time Native Image compilation, to generate a native executable of this Micronaut application.
Compiling Micronaut applications ahead of time with GraalVM significantly improves startup time and reduces the memory footprint of JVM-based applications.
Only Java and Kotlin projects support using GraalVM’s native-image tool. Groovy relies heavily on reflection, which is only partially supported by GraalVM.
|
8.1. GraalVM Installation
sdk install java 21.0.5-graal
For installation on Windows, or for a manual installation on Linux or Mac, see the GraalVM Getting Started documentation.
The previous command installs Oracle GraalVM, which is free to use in production and free to redistribute, at no cost, under the GraalVM Free Terms and Conditions.
Alternatively, you can use the GraalVM Community Edition:
sdk install java 21.0.2-graalce
8.2. Native Executable Generation
To generate a native executable using Gradle, run:
./gradlew nativeCompile
The native executable is created in build/native/nativeCompile
directory and can be run with build/native/nativeCompile/micronautguide
.
It is possible to customize the name of the native executable or pass additional parameters to GraalVM:
graalvmNative {
binaries {
main {
imageName.set('mn-graalvm-application') (1)
buildArgs.add('-Ob') (2)
}
}
}
1 | The native executable name will now be mn-graalvm-application |
2 | It is possible to pass extra build arguments to native-image . For example, -Ob enables the quick build mode. |
After you execute the native executable, navigate to localhost:8080 and authenticate with Okta.
9. Next Steps
Read Micronaut OAuth 2.0 documentation to learn more.
10. Help with the Micronaut Framework
The Micronaut Foundation sponsored the creation of this Guide. A variety of consulting and support services are available.
11. License
All guides are released with an Apache license 2.0 license for the code and a Creative Commons Attribution 4.0 license for the writing and media (images…). |