mn create-function-app example.micronaut.micronautguide --features=aws-lambda --build=gradle --lang=groovy
Deploy a Serverless Micronaut function to AWS Lambda Java 17 Runtime
Learn how to distribute a serverless Micronaut function to AWS Lambda 17 Runtime
Authors: Sergio del Amo
Micronaut Version: 4.6.3
Please read about Micronaut AWS Lambda Support to learn more about different Lambda runtime, Triggers, and Handlers, and how to integrate with a Micronaut application.
If you want to respond to triggers such as queue events, S3 events, or single endpoints, you should opt to code your Micronaut functions as Serverless functions.
In this guide, we will deploy a Micronaut serverless function to AWS Lambda.
1. Getting Started
In this guide, we will create a Micronaut application written in Groovy.
2. What you will need
To complete this guide, you will need the following:
-
Some time on your hands
-
A decent text editor or IDE (e.g. IntelliJ IDEA)
-
JDK 17 or greater installed with
JAVA_HOME
configured appropriately
3. Solution
We recommend that you follow the instructions in the next sections and create the application step by step. However, you can go right to the completed example.
-
Download and unzip the source
4. Writing the Application
Create an application using the Micronaut Command Line Interface or with Micronaut Launch.
If you don’t specify the --build argument, Gradle with the Kotlin DSL is used as the build tool. If you don’t specify the --lang argument, Java is used as the language.If you don’t specify the --test argument, JUnit is used for Java and Kotlin, and Spock is used for Groovy.
|
If you use Micronaut Launch, select serverless function as application type and add the aws-lambda
feature.
The previous command creates a Micronaut application with the default package example.micronaut
in a directory named micronautguide
.
The application contains a class extending MicronautRequestHandler
package example.micronaut
import io.micronaut.function.aws.MicronautRequestHandler
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import io.micronaut.json.JsonMapper;
import com.amazonaws.services.lambda.runtime.events.APIGatewayProxyRequestEvent
import com.amazonaws.services.lambda.runtime.events.APIGatewayProxyResponseEvent
import jakarta.inject.Inject
class FunctionRequestHandler extends MicronautRequestHandler<APIGatewayProxyRequestEvent, APIGatewayProxyResponseEvent> {
@Inject
JsonMapper objectMapper
@Override
APIGatewayProxyResponseEvent execute(APIGatewayProxyRequestEvent input) {
APIGatewayProxyResponseEvent response = new APIGatewayProxyResponseEvent()
try {
String json = new String(objectMapper.writeValueAsBytes([message: "Hello World"]))
response.statusCode = 200
response.body = json
} catch (IOException e) {
response.statusCode = 500
}
response
}
}
-
The class extends MicronautRequestHandler and defines input and output types.
An included test shows how to verify the function behaviour:
package example.micronaut
import com.amazonaws.services.lambda.runtime.events.APIGatewayProxyRequestEvent
import com.amazonaws.services.lambda.runtime.events.APIGatewayProxyResponseEvent
import spock.lang.AutoCleanup
import spock.lang.Shared
import spock.lang.Specification
class FunctionRequestHandlerSpec extends Specification {
@AutoCleanup
@Shared
FunctionRequestHandler handler = new FunctionRequestHandler()
void "test Handler"() {
given:
APIGatewayProxyRequestEvent request = new APIGatewayProxyRequestEvent()
request.setHttpMethod("GET")
request.setPath("/")
when:
APIGatewayProxyResponseEvent response = handler.execute(request)
then:
200 == response.getStatusCode().intValue()
"{\"message\":\"Hello World\"}" == response.body
}
}
-
When you instantiate the Handler, the application context starts.
-
Remember to close your application context when you end your test. You can use your handler to obtain it.
-
Invoke the
execute
method of the handler.
5. Testing the Application
To run the tests:
./gradlew test
Then open build/reports/tests/test/index.html
in a browser to see the results.
6. Lambda
Create a Lambda Function. As a runtime, select Java 17.
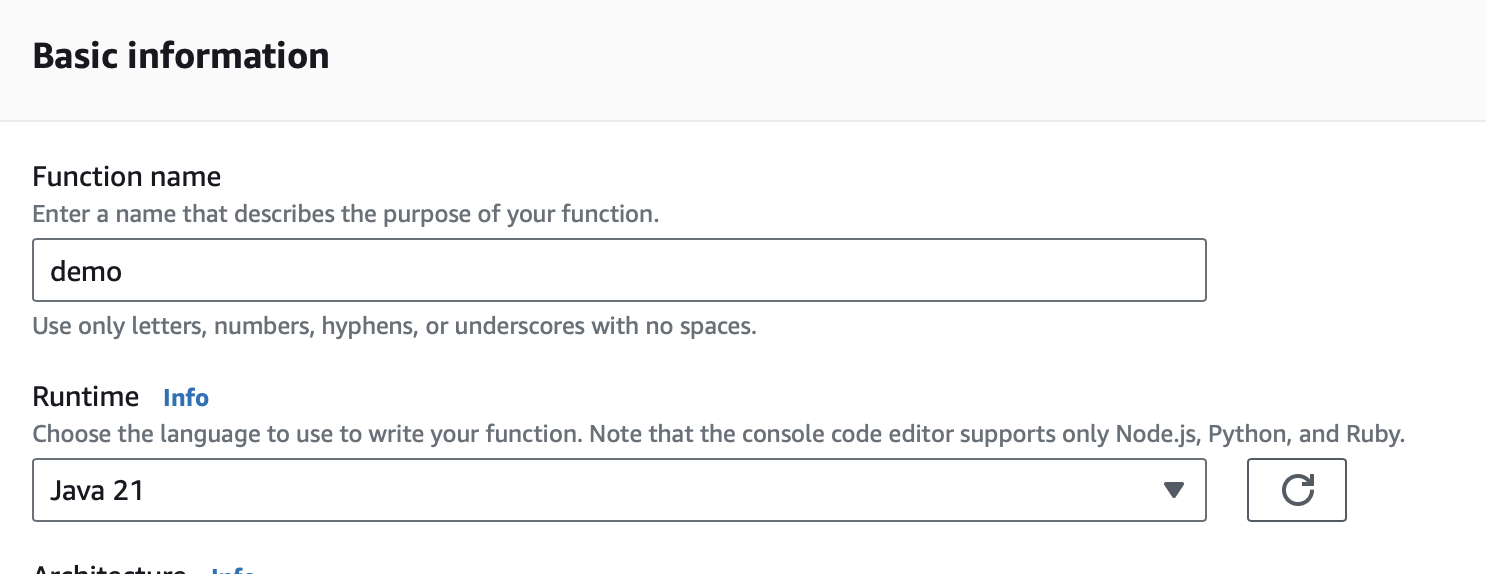
6.1. Upload Code
Create an executable jar including all dependencies:
./gradlew shadowJar
Upload it:
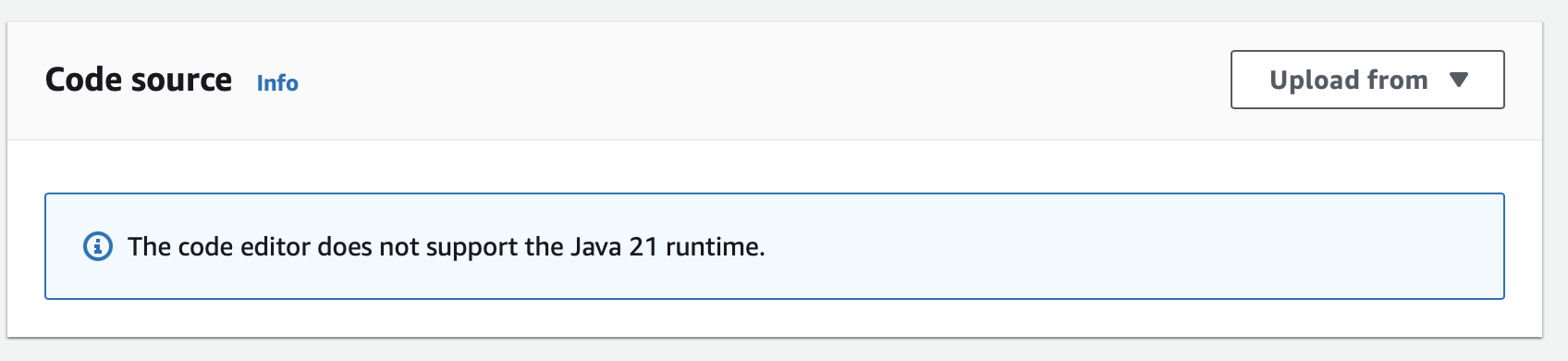
6.2. Handler
As Handler, set:
example.micronaut.FunctionRequestHandler

6.3. Test
You can test it easily with a JSON Event:
{
"path": "/",
"httpMethod": "GET",
"headers": {
"Accept": "application/json"
}
}
When working with Amazon API Gateway, it’s easy to use apigateway-aws-proxy
as an Event Template
to get started:
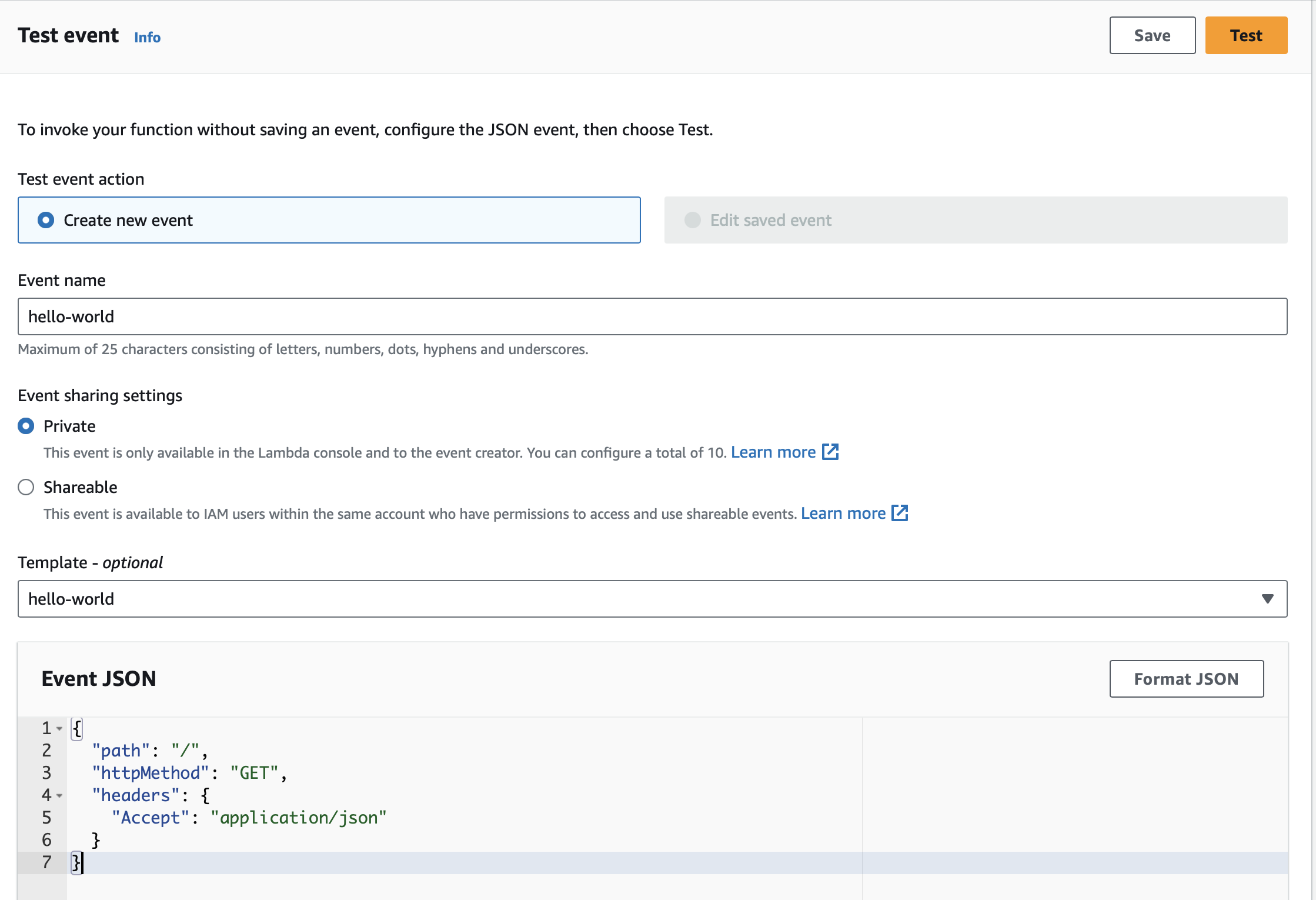
You should see a 200 response:
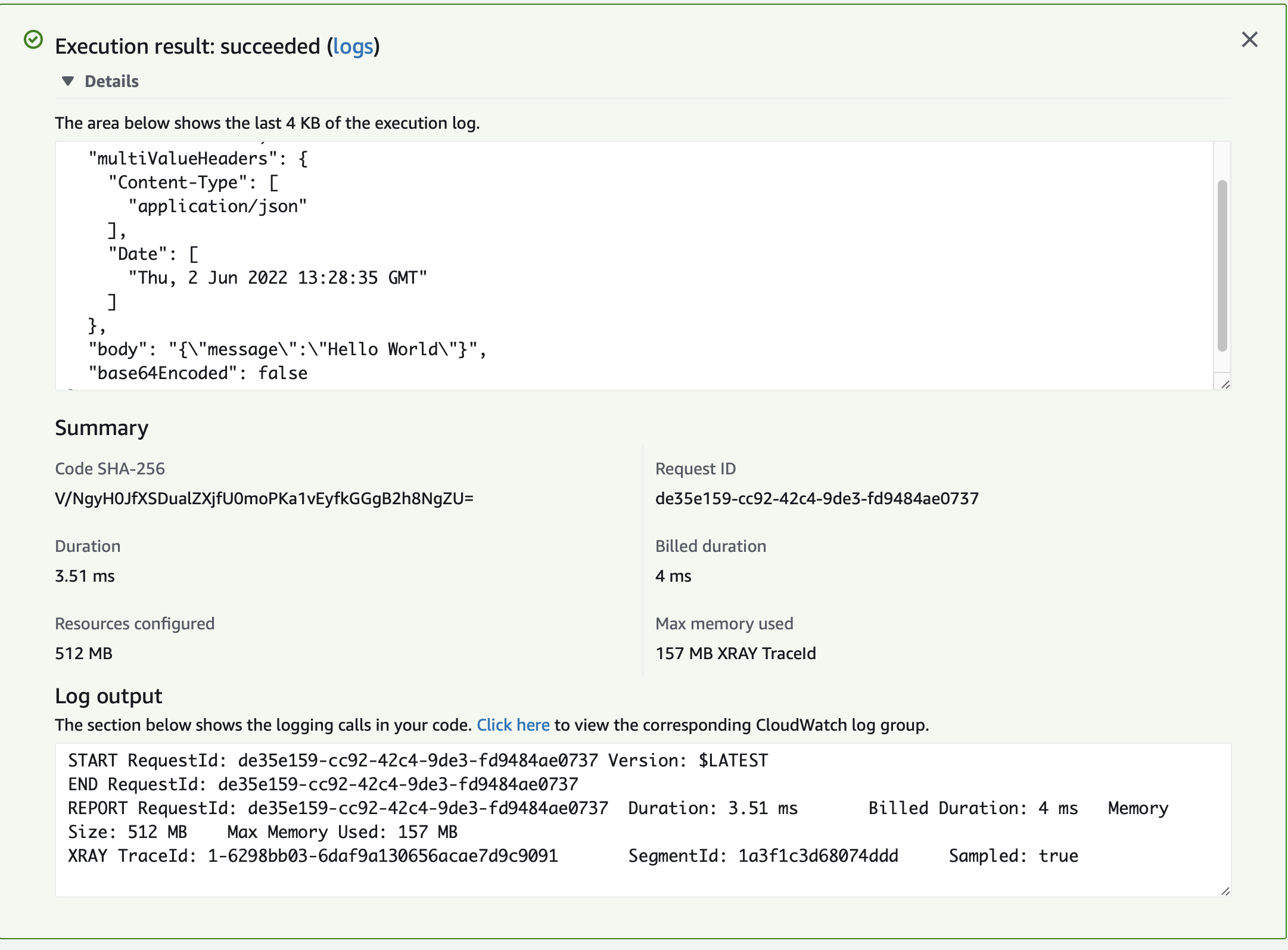
7. Next steps
Explore more features with Micronaut Guides.
Read more about:
8. Help with the Micronaut Framework
The Micronaut Foundation sponsored the creation of this Guide. A variety of consulting and support services are available.
9. License
All guides are released with an Apache license 2.0 license for the code and a Creative Commons Attribution 4.0 license for the writing and media (images…). |